Northing Calibration in FLASC#
Northing calibration, that is the detection of bias and changes in measurements of turbine yaw are important for many of the analysis in FLASC. This notebook demonstrates the use of several of these tools in FLASC for the calibration of northing measurements.
import warnings as wn
from datetime import timedelta as td
import numpy as np
import pandas as pd
from floris import TimeSeries
from floris.layout_visualization import plot_turbine_labels, plot_turbine_points
from floris.utilities import wrap_360
from matplotlib import pyplot as plt
from flasc import FlascDataFrame
from flasc.data_processing import (
dataframe_manipulations as dfm,
energy_ratio_wd_bias_estimation as best,
filtering as filt,
northing_offset as nof,
)
from flasc.data_processing.northing_offset_change_hoger import homogenize_hoger
from flasc.utilities import (
floris_tools as ftools,
optimization as flopt,
)
from flasc.utilities.utilities_examples import load_floris_artificial as load_floris
Load FLORIS model and show layout#
# Load FLORIS model
fm, turbine_weights = load_floris()
# Show the layout
fig, ax = plt.subplots()
plot_turbine_points(fm, ax)
plot_turbine_labels(fm, ax)
<Axes: >
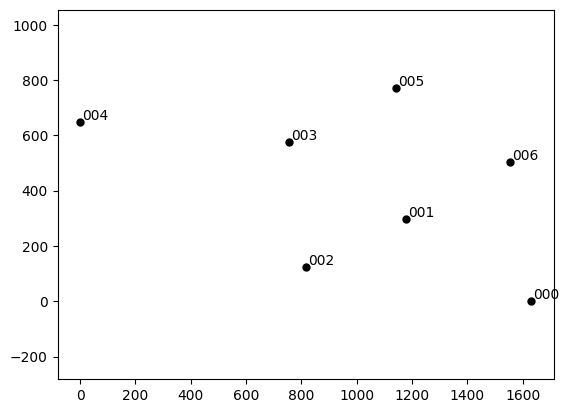
Generate data set to illustrate operations#
For simplicity assume a fixed wind speed and turbulence intensity and uniform wind direction. Perturb the wind direction by random noise
# Set wind direction to step from 90 to 270, in steps that are 600 long and 10 degrees wide
wind_directions = np.linspace(90, 270, 10)
wind_directions = np.tile(wind_directions, 600)
wind_directions = np.sort(wind_directions)
# Apply noise
np.random.seed(0)
noise = np.random.normal(0, 5.0, wind_directions.shape)
wind_directions = wind_directions + noise
# Set a FLORIS time series object
time_series = TimeSeries(
wind_directions=wind_directions, wind_speeds=8.0, turbulence_intensities=0.06
)
# Calculate FLORIS solution
fm.set(wind_data=time_series)
fm.run()
turbine_powers = fm.get_turbine_powers()
# Add random noise to the power output
turbine_powers = turbine_powers + np.random.normal(0, 25.0, turbine_powers.shape)
# Use the results to create a FLASC dataframe representing hypothetical scada data
df_scada = FlascDataFrame(
{
"time": pd.date_range(start="1/1/2020", periods=len(wind_directions), freq="600s"),
"wind_directions": wind_directions,
"wind_speeds": 8.0 * np.ones_like(wind_directions),
"ti": 0.06 * np.ones_like(wind_directions),
}
)
# Add the turbine powers to the dataframe with some added noise
for t_idx in range(fm.n_turbines):
df_scada[f"pow_{t_idx:03d}"] = turbine_powers[:, t_idx]
# Set the turbine wind directions to be the true wind direction with some added noise
for t_idx in range(fm.n_turbines):
df_scada[f"wd_{t_idx:03d}"] = wrap_360(
wind_directions + np.random.normal(0, 5.0, wind_directions.shape)
)
# Set wind speeds to be fixed with small noise
for t_idx in range(fm.n_turbines):
df_scada[f"ws_{t_idx:03d}"] = 8.0 * np.ones_like(wind_directions) + np.random.normal(
0, 0.1, wind_directions.shape
)
df_scada
time | wind_directions | wind_speeds | ti | pow_000 | pow_001 | pow_002 | pow_003 | pow_004 | pow_005 | ... | wd_004 | wd_005 | wd_006 | ws_000 | ws_001 | ws_002 | ws_003 | ws_004 | ws_005 | ws_006 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 2020-01-01 00:00:00 | 98.820262 | 8.0 | 0.06 | 1.754006e+06 | 1.753879e+06 | 6.357473e+05 | 9.640375e+05 | 9.666355e+05 | 1.753524e+06 | ... | 99.644321 | 101.107205 | 99.348930 | 8.011852 | 8.162351 | 8.159744 | 7.899556 | 8.076833 | 8.185659 | 8.064289 |
1 | 2020-01-01 00:10:00 | 92.000786 | 8.0 | 0.06 | 1.753913e+06 | 1.753904e+06 | 1.409497e+06 | 8.553318e+05 | 9.171484e+05 | 1.753951e+06 | ... | 82.166085 | 88.043464 | 89.257707 | 8.175628 | 7.808788 | 8.023940 | 8.084883 | 8.080681 | 7.849506 | 7.913113 |
2 | 2020-01-01 00:20:00 | 94.893690 | 8.0 | 0.06 | 1.753985e+06 | 1.753891e+06 | 9.842584e+05 | 6.162996e+05 | 6.600864e+05 | 1.753942e+06 | ... | 94.712330 | 92.252229 | 90.985282 | 7.991109 | 7.892661 | 7.928803 | 8.081048 | 8.073745 | 8.091502 | 8.053268 |
3 | 2020-01-01 00:30:00 | 101.204466 | 8.0 | 0.06 | 1.753950e+06 | 1.753555e+06 | 8.123594e+05 | 1.319409e+06 | 1.170321e+06 | 1.751776e+06 | ... | 110.699134 | 93.116417 | 108.537493 | 7.917771 | 8.077458 | 7.942418 | 7.960415 | 7.766181 | 7.875381 | 8.012211 |
4 | 2020-01-01 00:40:00 | 99.337790 | 8.0 | 0.06 | 1.754020e+06 | 1.753852e+06 | 6.487232e+05 | 1.043126e+06 | 1.030381e+06 | 1.753318e+06 | ... | 95.656436 | 92.461185 | 106.783658 | 7.850214 | 7.973655 | 8.028457 | 8.225742 | 8.147089 | 7.934316 | 7.798261 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
5995 | 2020-02-11 15:10:00 | 263.345981 | 8.0 | 0.06 | 1.753204e+06 | 1.687681e+06 | 1.753965e+06 | 1.730570e+06 | 1.753947e+06 | 8.849585e+05 | ... | 261.464863 | 265.654828 | 265.006674 | 7.867506 | 8.118957 | 7.879889 | 7.999180 | 8.046216 | 7.930540 | 7.880831 |
5996 | 2020-02-11 15:20:00 | 271.762993 | 8.0 | 0.06 | 1.435174e+06 | 1.752561e+06 | 1.753922e+06 | 9.033320e+05 | 1.753985e+06 | 1.702120e+06 | ... | 271.559170 | 267.387924 | 271.051594 | 8.028336 | 8.219887 | 7.878284 | 8.086875 | 8.154903 | 8.149477 | 8.080819 |
5997 | 2020-02-11 15:30:00 | 267.310576 | 8.0 | 0.06 | 1.728385e+06 | 1.740995e+06 | 1.753938e+06 | 1.521491e+06 | 1.753960e+06 | 1.223109e+06 | ... | 267.398639 | 263.998493 | 270.306531 | 8.020642 | 8.044316 | 8.031791 | 8.170447 | 8.253171 | 8.056111 | 7.903335 |
5998 | 2020-02-11 15:40:00 | 271.967222 | 8.0 | 0.06 | 1.408887e+06 | 1.752647e+06 | 1.753962e+06 | 8.717409e+05 | 1.753956e+06 | 1.709693e+06 | ... | 270.043104 | 273.116864 | 281.039771 | 8.063863 | 7.936743 | 8.230575 | 8.071727 | 7.996428 | 8.013610 | 8.109070 |
5999 | 2020-02-11 15:50:00 | 271.432591 | 8.0 | 0.06 | 1.475155e+06 | 1.752267e+06 | 1.753954e+06 | 9.545584e+05 | 1.753887e+06 | 1.687766e+06 | ... | 269.690265 | 269.465325 | 269.938743 | 7.972016 | 8.079571 | 8.001622 | 8.017239 | 8.017320 | 8.013297 | 7.983608 |
6000 rows × 25 columns
Northing calibration error#
Add to the data two types of northing calibration error:
A constant bias on turbine 001
A change in bias on turbine 002 halfway through the data set
df_scada["wd_001"] = wrap_360(
15.0 + wind_directions + np.random.normal(0, 0.5, wind_directions.shape)
)
mid_point = int(len(wind_directions) / 2)
wd_change = wind_directions + np.random.normal(0, 0.5, wind_directions.shape)
wd_change[mid_point:] = wd_change[mid_point:] - 45.0
wd_change = wrap_360(wd_change)
df_scada["wd_002"] = wd_change
# Show the wd channels for the turbines
fig, ax = plt.subplots(figsize=(12, 6))
ax.plot(df_scada["time"], df_scada["wd_001"], label="wd_001 (Fixed Bias)", color="blue")
ax.plot(df_scada["time"], df_scada["wd_002"], label="wd_002 (Bias Changes)", color="red")
ax.plot(df_scada["time"], df_scada["wd_000"], label="wd_000", color="k", alpha=0.5)
ax.plot(df_scada["time"], df_scada["wd_003"], label="wd_003", color="k", alpha=0.5)
ax.plot(df_scada["time"], df_scada["wd_004"], label="wd_004", color="k", alpha=0.5)
ax.plot(df_scada["time"], df_scada["wd_005"], label="wd_005", color="k", alpha=0.5)
ax.plot(df_scada["time"], df_scada["wd_006"], label="wd_006", color="k", alpha=0.5)
ax.legend()
ax.set_xlabel("Time")
ax.set_ylabel("Wind direction (deg)")
Text(0, 0.5, 'Wind direction (deg)')
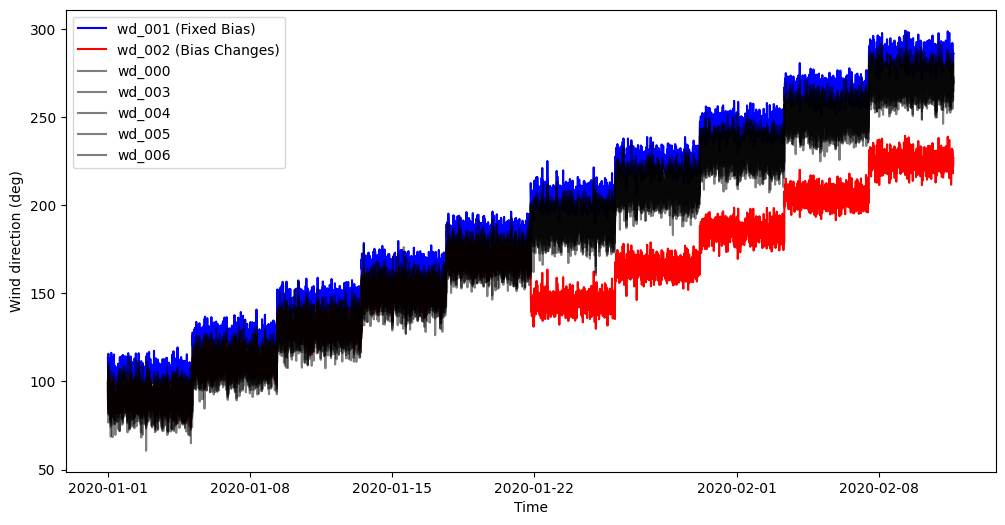
# Finally compute df_approx for use in later algorithms
# Can compute only at 8m/s for this example
df_fm_approx = ftools.calc_floris_approx_table(
fm=fm, # fi=fi_pci,
wd_array=np.arange(0.0, 360.01, 1.0),
ws_array=np.array([8.0]),
ti_array=np.array([0.06]),
)
2025-03-04 21:48:17 Generating a df_approx table of FLORIS solutions covering a total of 361 cases.
2025-03-04 21:48:18 Finished calculating the FLORIS solutions for the dataframe.
Cross-Check Northing calibration#
crosscheck_northing_offset_consistency
is a function to check if the relative offset between turbines is consistent. If the offset is consistent, then we know we can find a single offset value that would align the two turbine's northings. If this is not the case, one or both turbines likely experience jumps in their nacelle calibration throughout the timeseries.
# Create a copy in which we mark the wd measurements of turbines with northing drift as faulty
df_scada_marked_faulty_northing_drift = df_scada.copy()
turb_wd_consistency = nof.crosscheck_northing_offset_consistency(
df=df_scada_marked_faulty_northing_drift, fm=fm, plot_figure=True, bias_timestep=td(days=1)
)
print(turb_wd_consistency)
2025-03-04 21:48:18 Matching curves for turbine 000...
2025-03-04 21:48:19 T006 T001 T002 T005 T003
0 0.0 -16.0 0.0 0.0 0.0
1 0.0 -14.0 0.0 0.0 0.0
2 0.0 -16.0 0.0 0.0 0.0
3 0.0 -16.0 0.0 0.0 0.0
4 0.0 -14.0 0.0 2.0 0.0
5 0.0 -14.0 0.0 0.0 0.0
6 0.0 -16.0 0.0 0.0 -2.0
7 0.0 -16.0 0.0 0.0 0.0
8 0.0 -16.0 0.0 0.0 0.0
9 0.0 -16.0 0.0 0.0 0.0
10 0.0 -16.0 0.0 0.0 0.0
11 0.0 -16.0 0.0 0.0 0.0
12 0.0 -14.0 0.0 0.0 0.0
13 0.0 -14.0 0.0 0.0 0.0
14 -2.0 -16.0 0.0 0.0 0.0
15 0.0 -14.0 0.0 0.0 0.0
16 2.0 -14.0 0.0 2.0 0.0
17 0.0 -14.0 0.0 0.0 0.0
18 -2.0 -16.0 0.0 0.0 0.0
19 0.0 -16.0 0.0 0.0 0.0
20 0.0 -14.0 8.0 0.0 2.0
21 0.0 -14.0 46.0 0.0 0.0
22 0.0 -14.0 46.0 0.0 0.0
23 0.0 -14.0 46.0 0.0 0.0
24 0.0 -14.0 46.0 2.0 0.0
25 0.0 -16.0 44.0 0.0 0.0
26 0.0 -16.0 44.0 -2.0 0.0
27 0.0 -14.0 46.0 0.0 2.0
28 0.0 -14.0 46.0 0.0 0.0
29 0.0 -14.0 46.0 0.0 0.0
30 0.0 -14.0 44.0 0.0 0.0
31 0.0 -16.0 44.0 0.0 0.0
32 0.0 -14.0 46.0 0.0 0.0
33 0.0 -16.0 44.0 0.0 0.0
34 0.0 -14.0 46.0 0.0 0.0
35 0.0 -16.0 44.0 0.0 0.0
36 0.0 -14.0 46.0 0.0 2.0
37 0.0 -14.0 46.0 0.0 0.0
38 0.0 -14.0 46.0 0.0 0.0
39 0.0 -14.0 46.0 0.0 0.0
40 0.0 -16.0 44.0 0.0 0.0
41 0.0 -14.0 46.0 0.0 0.0
2025-03-04 21:48:19 Matching curves for turbine 001...
2025-03-04 21:48:20 T002 T006 T005 T003 T000
0 16.0 14.0 16.0 16.0 16.0
1 14.0 16.0 14.0 16.0 14.0
2 14.0 16.0 14.0 16.0 16.0
3 16.0 16.0 14.0 14.0 16.0
4 14.0 14.0 16.0 14.0 14.0
5 16.0 14.0 14.0 16.0 14.0
6 14.0 14.0 14.0 14.0 16.0
7 16.0 14.0 16.0 16.0 16.0
8 14.0 16.0 14.0 16.0 16.0
9 14.0 14.0 14.0 16.0 16.0
10 16.0 14.0 14.0 14.0 16.0
11 16.0 14.0 16.0 14.0 16.0
12 16.0 14.0 14.0 14.0 14.0
13 14.0 14.0 14.0 14.0 14.0
14 16.0 14.0 16.0 16.0 16.0
15 14.0 14.0 14.0 16.0 14.0
16 16.0 16.0 16.0 14.0 14.0
17 14.0 14.0 14.0 16.0 14.0
18 16.0 14.0 14.0 16.0 16.0
19 16.0 14.0 14.0 14.0 16.0
20 22.0 14.0 14.0 16.0 14.0
21 60.0 14.0 16.0 14.0 14.0
22 60.0 14.0 16.0 14.0 14.0
23 60.0 14.0 16.0 16.0 14.0
24 60.0 16.0 16.0 14.0 14.0
25 60.0 16.0 14.0 16.0 16.0
26 60.0 16.0 14.0 14.0 16.0
27 60.0 14.0 16.0 16.0 14.0
28 60.0 14.0 16.0 14.0 14.0
29 60.0 14.0 16.0 16.0 14.0
30 60.0 14.0 14.0 14.0 14.0
31 60.0 16.0 14.0 14.0 16.0
32 60.0 16.0 16.0 14.0 14.0
33 60.0 16.0 16.0 16.0 16.0
34 60.0 16.0 16.0 16.0 14.0
35 60.0 14.0 16.0 14.0 16.0
36 60.0 14.0 14.0 16.0 14.0
37 60.0 14.0 16.0 14.0 14.0
38 60.0 16.0 14.0 14.0 14.0
39 60.0 14.0 16.0 14.0 14.0
40 60.0 16.0 14.0 14.0 16.0
41 60.0 14.0 16.0 14.0 14.0
2025-03-04 21:48:20 Matching curves for turbine 002...
2025-03-04 21:48:21 T001 T003 T005 T000 T006
0 -16.0 0.0 0.0 -0.0 0.0
1 -14.0 0.0 0.0 -0.0 0.0
2 -14.0 0.0 0.0 -0.0 0.0
3 -16.0 0.0 0.0 -0.0 0.0
4 -14.0 0.0 0.0 -0.0 0.0
5 -16.0 0.0 0.0 -0.0 0.0
6 -14.0 0.0 0.0 -0.0 0.0
7 -16.0 0.0 0.0 -0.0 0.0
8 -14.0 0.0 0.0 -0.0 0.0
9 -14.0 0.0 0.0 -0.0 0.0
10 -16.0 0.0 0.0 -0.0 0.0
11 -16.0 0.0 0.0 -0.0 0.0
12 -16.0 0.0 0.0 -0.0 0.0
13 -14.0 0.0 0.0 -0.0 0.0
14 -16.0 0.0 0.0 -0.0 0.0
15 -14.0 0.0 0.0 -0.0 0.0
16 -16.0 0.0 2.0 -0.0 0.0
17 -14.0 0.0 0.0 -0.0 0.0
18 -16.0 0.0 0.0 -0.0 0.0
19 -16.0 0.0 0.0 -0.0 0.0
20 -22.0 -6.0 -8.0 -8.0 -8.0
21 -60.0 -46.0 -44.0 -46.0 -46.0
22 -60.0 -46.0 -44.0 -46.0 -46.0
23 -60.0 -44.0 -44.0 -46.0 -46.0
24 -60.0 -46.0 -44.0 -46.0 -44.0
25 -60.0 -44.0 -46.0 -44.0 -44.0
26 -60.0 -46.0 -46.0 -44.0 -44.0
27 -60.0 -44.0 -44.0 -46.0 -46.0
28 -60.0 -46.0 -44.0 -46.0 -46.0
29 -60.0 -44.0 -44.0 -46.0 -46.0
30 -60.0 -46.0 -46.0 -44.0 -46.0
31 -60.0 -46.0 -46.0 -44.0 -44.0
32 -60.0 -46.0 -44.0 -46.0 -44.0
33 -60.0 -44.0 -44.0 -44.0 -44.0
34 -60.0 -44.0 -44.0 -46.0 -44.0
35 -60.0 -46.0 -44.0 -44.0 -46.0
36 -60.0 -44.0 -46.0 -46.0 -46.0
37 -60.0 -46.0 -44.0 -46.0 -44.0
38 -60.0 -46.0 -46.0 -46.0 -44.0
39 -60.0 -46.0 -44.0 -46.0 -46.0
40 -60.0 -46.0 -46.0 -44.0 -44.0
41 -60.0 -46.0 -44.0 -46.0 -46.0
2025-03-04 21:48:21 Matching curves for turbine 003...
2025-03-04 21:48:22 T005 T002 T001 T004 T006
0 0.0 -0.0 -16.0 0.0 0.0
1 0.0 -0.0 -16.0 0.0 0.0
2 0.0 -0.0 -16.0 0.0 0.0
3 0.0 -0.0 -14.0 0.0 0.0
4 0.0 -0.0 -14.0 0.0 0.0
5 0.0 -0.0 -16.0 0.0 0.0
6 0.0 -0.0 -14.0 0.0 0.0
7 0.0 -0.0 -16.0 0.0 0.0
8 0.0 -0.0 -16.0 0.0 0.0
9 0.0 -0.0 -16.0 0.0 0.0
10 0.0 -0.0 -14.0 0.0 0.0
11 0.0 -0.0 -14.0 0.0 0.0
12 0.0 -0.0 -14.0 0.0 0.0
13 0.0 -0.0 -14.0 0.0 0.0
14 0.0 -0.0 -16.0 0.0 0.0
15 0.0 -0.0 -16.0 0.0 0.0
16 2.0 -0.0 -14.0 2.0 2.0
17 0.0 -0.0 -16.0 0.0 0.0
18 0.0 -0.0 -16.0 0.0 0.0
19 0.0 -0.0 -14.0 0.0 0.0
20 -2.0 6.0 -16.0 0.0 -2.0
21 0.0 46.0 -14.0 0.0 0.0
22 0.0 46.0 -14.0 0.0 0.0
23 0.0 44.0 -16.0 0.0 0.0
24 0.0 46.0 -14.0 0.0 0.0
25 0.0 44.0 -16.0 -2.0 0.0
26 0.0 46.0 -14.0 0.0 0.0
27 0.0 44.0 -16.0 0.0 0.0
28 0.0 46.0 -14.0 0.0 0.0
29 0.0 44.0 -16.0 -2.0 0.0
30 0.0 46.0 -14.0 0.0 0.0
31 0.0 46.0 -14.0 0.0 0.0
32 0.0 46.0 -14.0 0.0 0.0
33 0.0 44.0 -16.0 0.0 0.0
34 0.0 44.0 -16.0 0.0 0.0
35 0.0 46.0 -14.0 0.0 0.0
36 -2.0 44.0 -16.0 -2.0 -2.0
37 0.0 46.0 -14.0 0.0 0.0
38 0.0 46.0 -14.0 0.0 0.0
39 0.0 46.0 -14.0 0.0 0.0
40 0.0 46.0 -14.0 0.0 0.0
41 0.0 46.0 -14.0 0.0 0.0
2025-03-04 21:48:22 Matching curves for turbine 004...
2025-03-04 21:48:23 T003 T002 T005 T001 T006
0 -0.0 0.0 0.0 -16.0 0.0
1 -0.0 0.0 0.0 -14.0 0.0
2 -0.0 0.0 0.0 -16.0 0.0
3 -0.0 0.0 0.0 -16.0 0.0
4 -0.0 0.0 0.0 -16.0 -2.0
5 -0.0 0.0 0.0 -14.0 0.0
6 -0.0 0.0 0.0 -16.0 0.0
7 -0.0 0.0 0.0 -14.0 0.0
8 -0.0 0.0 0.0 -14.0 0.0
9 -0.0 0.0 0.0 -16.0 0.0
10 -0.0 0.0 0.0 -16.0 -2.0
11 -0.0 0.0 0.0 -14.0 0.0
12 -0.0 0.0 0.0 -16.0 0.0
13 -0.0 0.0 0.0 -16.0 0.0
14 -0.0 0.0 0.0 -16.0 0.0
15 -0.0 0.0 0.0 -16.0 0.0
16 -2.0 0.0 0.0 -16.0 0.0
17 -0.0 0.0 0.0 -16.0 0.0
18 -0.0 0.0 0.0 -14.0 0.0
19 -0.0 0.0 0.0 -14.0 0.0
20 -0.0 8.0 0.0 -14.0 0.0
21 -0.0 44.0 0.0 -16.0 0.0
22 -0.0 44.0 0.0 -16.0 0.0
23 -0.0 46.0 0.0 -14.0 0.0
24 -0.0 46.0 2.0 -14.0 0.0
25 2.0 46.0 0.0 -14.0 2.0
26 -0.0 44.0 -2.0 -16.0 0.0
27 -0.0 46.0 0.0 -14.0 0.0
28 -0.0 44.0 0.0 -16.0 0.0
29 2.0 46.0 2.0 -14.0 0.0
30 -0.0 46.0 0.0 -14.0 0.0
31 -0.0 44.0 -2.0 -16.0 0.0
32 -0.0 46.0 0.0 -14.0 0.0
33 -0.0 46.0 0.0 -14.0 0.0
34 -0.0 44.0 0.0 -16.0 0.0
35 -0.0 46.0 0.0 -14.0 0.0
36 2.0 46.0 0.0 -14.0 0.0
37 -0.0 46.0 0.0 -14.0 0.0
38 -0.0 44.0 0.0 -16.0 0.0
39 -0.0 44.0 0.0 -16.0 0.0
40 -0.0 44.0 0.0 -16.0 0.0
41 -0.0 46.0 0.0 -14.0 0.0
2025-03-04 21:48:23 Matching curves for turbine 005...
2025-03-04 21:48:23 T003 T001 T006 T002 T000
0 -0.0 -16.0 0.0 -0.0 -0.0
1 -0.0 -14.0 0.0 -0.0 -0.0
2 -0.0 -14.0 0.0 -0.0 -0.0
3 -0.0 -14.0 0.0 -0.0 -0.0
4 -0.0 -16.0 -2.0 -0.0 -2.0
5 -0.0 -14.0 0.0 -0.0 -0.0
6 -0.0 -14.0 0.0 -0.0 -0.0
7 -0.0 -16.0 0.0 -0.0 -0.0
8 -0.0 -14.0 0.0 -0.0 -0.0
9 -0.0 -14.0 0.0 -0.0 -0.0
10 -0.0 -14.0 0.0 -0.0 -0.0
11 -0.0 -16.0 0.0 -0.0 -0.0
12 -0.0 -14.0 0.0 -0.0 -0.0
13 -0.0 -14.0 0.0 -0.0 -0.0
14 -0.0 -16.0 -2.0 -0.0 -0.0
15 -0.0 -14.0 0.0 -0.0 -0.0
16 -2.0 -16.0 0.0 -2.0 -2.0
17 -0.0 -14.0 0.0 -0.0 -0.0
18 -0.0 -14.0 0.0 -0.0 -0.0
19 -0.0 -14.0 0.0 -0.0 -0.0
20 2.0 -14.0 0.0 8.0 -0.0
21 -0.0 -16.0 0.0 44.0 -0.0
22 -0.0 -16.0 -2.0 44.0 -0.0
23 -0.0 -16.0 0.0 44.0 -0.0
24 -0.0 -16.0 0.0 44.0 -2.0
25 -0.0 -14.0 0.0 46.0 -0.0
26 -0.0 -14.0 2.0 46.0 2.0
27 -0.0 -16.0 0.0 44.0 -0.0
28 -0.0 -16.0 0.0 44.0 -0.0
29 -0.0 -16.0 0.0 44.0 -0.0
30 -0.0 -14.0 0.0 46.0 -0.0
31 -0.0 -14.0 0.0 46.0 -0.0
32 -0.0 -16.0 0.0 44.0 -0.0
33 -0.0 -16.0 0.0 44.0 -0.0
34 -0.0 -16.0 0.0 44.0 -0.0
35 -0.0 -16.0 0.0 44.0 -0.0
36 2.0 -14.0 0.0 46.0 -0.0
37 -0.0 -16.0 0.0 44.0 -0.0
38 -0.0 -14.0 0.0 46.0 -0.0
39 -0.0 -16.0 0.0 44.0 -0.0
40 -0.0 -14.0 0.0 46.0 -0.0
41 -0.0 -16.0 -2.0 44.0 -0.0
2025-03-04 21:48:23 Matching curves for turbine 006...
2025-03-04 21:48:23 T001 T005 T000 T003 T002
0 -14.0 -0.0 -0.0 -0.0 -0.0
1 -16.0 -0.0 -0.0 -0.0 -0.0
2 -16.0 -0.0 -0.0 -0.0 -0.0
3 -16.0 -0.0 -0.0 -0.0 -0.0
4 -14.0 2.0 -0.0 -0.0 -0.0
5 -14.0 -0.0 -0.0 -0.0 -0.0
6 -14.0 -0.0 -0.0 -0.0 -0.0
7 -14.0 -0.0 -0.0 -0.0 -0.0
8 -16.0 -0.0 -0.0 -0.0 -0.0
9 -14.0 -0.0 -0.0 -0.0 -0.0
10 -14.0 -0.0 -0.0 -0.0 -0.0
11 -14.0 -0.0 -0.0 -0.0 -0.0
12 -14.0 -0.0 -0.0 -0.0 -0.0
13 -14.0 -0.0 -0.0 -0.0 -0.0
14 -14.0 2.0 2.0 -0.0 -0.0
15 -14.0 -0.0 -0.0 -0.0 -0.0
16 -16.0 -0.0 -2.0 -2.0 -0.0
17 -14.0 -0.0 -0.0 -0.0 -0.0
18 -14.0 -0.0 2.0 -0.0 -0.0
19 -14.0 -0.0 -0.0 -0.0 -0.0
20 -14.0 -0.0 -0.0 2.0 8.0
21 -14.0 -0.0 -0.0 -0.0 46.0
22 -14.0 2.0 -0.0 -0.0 46.0
23 -14.0 -0.0 -0.0 -0.0 46.0
24 -16.0 -0.0 -0.0 -0.0 44.0
25 -16.0 -0.0 -0.0 -0.0 44.0
26 -16.0 -2.0 -0.0 -0.0 44.0
27 -14.0 -0.0 -0.0 -0.0 46.0
28 -14.0 -0.0 -0.0 -0.0 46.0
29 -14.0 -0.0 -0.0 -0.0 46.0
30 -14.0 -0.0 -0.0 -0.0 46.0
31 -16.0 -0.0 -0.0 -0.0 44.0
32 -16.0 -0.0 -0.0 -0.0 44.0
33 -16.0 -0.0 -0.0 -0.0 44.0
34 -16.0 -0.0 -0.0 -0.0 44.0
35 -14.0 -0.0 -0.0 -0.0 46.0
36 -14.0 -0.0 -0.0 2.0 46.0
37 -14.0 -0.0 -0.0 -0.0 44.0
38 -16.0 -0.0 -0.0 -0.0 44.0
39 -14.0 -0.0 -0.0 -0.0 46.0
40 -16.0 -0.0 -0.0 -0.0 44.0
41 -14.0 2.0 -0.0 -0.0 46.0
2025-03-04 21:48:23 Turbine 000 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:23 Turbine 001 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:23 Turbine 002 seems to have one or multiple jumps in its WD measurement calibration. [BAD]
2025-03-04 21:48:23 Turbine 003 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:23 Turbine 004 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:23 Turbine 005 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:23 Turbine 006 seems to have no jumps in its WD measurement calibration. [CLEAN]
['clean', 'clean', 'bad', 'clean', 'clean', 'clean', 'clean']
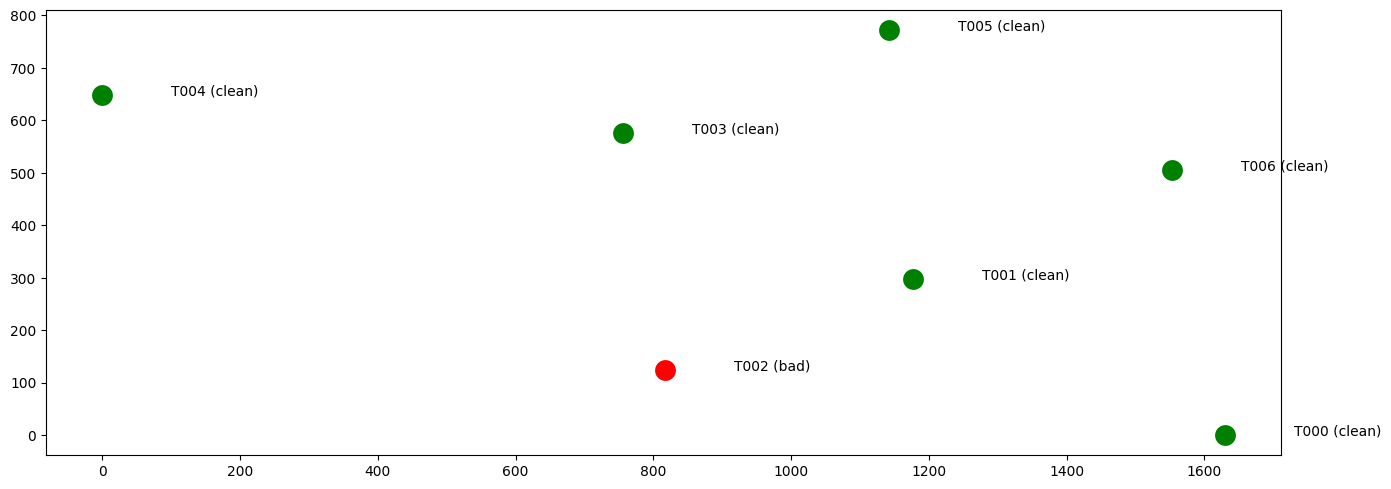
crosscheck_northing_offset_consistency
detects that T002 contains a probable jump, one solution is to then remove T002's wind direction data from consideration however this is not done in this notebook as we next take advantage of HOGER recalibration. The code to do this is included below in comments
# # Mark wind direction measurements of turbines with inconsistent calibration as faulty
# faulty_turbines = [not s == "clean" for s in turb_wd_consistency]
# for ti in np.where(faulty_turbines)[0]:
# df_scada_marked_faulty_northing_drift["wd_{:03d}".format(ti)] = np.nan
Homegenization with HOGER#
The homogenize
function implements the HOGER method for recalibrating northing measurements. HOGER was developed by Paul Poncet (engie-paul-poncet)
and Thomas Duc (engie-thomas-duc) of Engie, and Rubén González-Lope (rglope) and Alvaro Gonzalez Salcedo (alvarogonzalezsalcedo) of CENER within the TWAIN project.
The homogenize
will remove apparent jumps in northing correction (but does not confirm the final level is unbiased overall)
df_scada_non_homogenized = df_scada.copy()
df_scada_homogenized, d2 = homogenize_hoger(df_scada_marked_faulty_northing_drift, threshold=10)
# Show the search results
d2
[2999.5]
/home/runner/work/flasc/flasc/flasc/data_processing/northing_offset_change_hoger.py:110: UserWarning: Encountered a tie, and the difference between minimal and maximal value is > length('x') * 0.05.
The distribution could be multimodal
warnings.warn(
Class | Turbine | Count | Jump | Knot | Knot_date | |
---|---|---|---|---|---|---|
0 | 1 | wd_002 | 6 | 44.982446 | 2999.5 | 2020-01-21 19:40:00 |
The HOGER homeginization removes changes in northing calibration of turbines with respect to others, however the final selected value may contain a steady offset, as happens in this case
# Show the effects of homogenization
# Show the wd channels for the turbines
fig, ax = plt.subplots(figsize=(12, 6))
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_001"],
label="wd_001 (Fixed Bias)",
color="blue",
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_002"],
label="wd_002 (Bias Changes)",
color="red",
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_000"],
label="wd_000",
color="k",
alpha=0.5,
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_003"],
label="wd_003",
color="k",
alpha=0.5,
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_004"],
label="wd_004",
color="k",
alpha=0.5,
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_005"],
label="wd_005",
color="k",
alpha=0.5,
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_006"],
label="wd_006",
color="k",
alpha=0.5,
)
ax.legend()
ax.set_xlabel("Time")
ax.set_ylabel("Wind direction (deg)")
Text(0, 0.5, 'Wind direction (deg)')
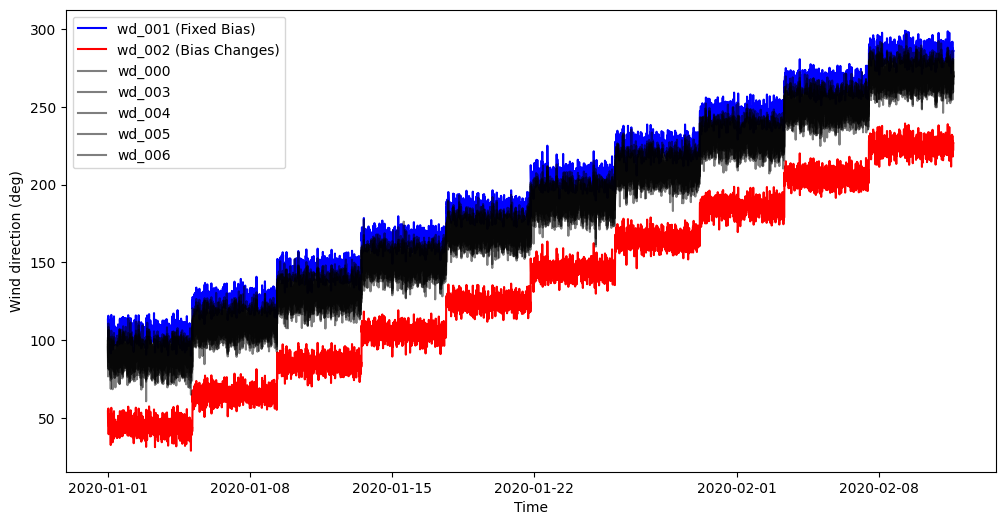
Recalculate which turbines are clean with respect to offset changes#
# Create a copy in which we mark the wd measurements of turbines with northing drift as faulty
df_scada_marked_faulty_northing_drift = df_scada_homogenized.copy()
turb_wd_consistency = nof.crosscheck_northing_offset_consistency(
df=df_scada_marked_faulty_northing_drift, fm=fm, plot_figure=True, bias_timestep=td(days=2)
)
print(turb_wd_consistency)
2025-03-04 21:48:24 Matching curves for turbine 000...
2025-03-04 21:48:24 T006 T001 T002 T005 T003
0 0.0 -16.0 44.0 0.0 0.0
1 0.0 -16.0 44.0 0.0 0.0
2 0.0 -14.0 46.0 0.0 0.0
3 0.0 -16.0 44.0 0.0 0.0
4 0.0 -16.0 44.0 0.0 0.0
5 0.0 -16.0 44.0 0.0 0.0
6 0.0 -14.0 46.0 0.0 0.0
7 0.0 -16.0 44.0 0.0 0.0
8 0.0 -14.0 46.0 0.0 0.0
9 0.0 -16.0 44.0 0.0 0.0
10 0.0 -14.0 46.0 0.0 0.0
11 0.0 -14.0 46.0 0.0 0.0
12 0.0 -14.0 44.0 0.0 0.0
13 0.0 -14.0 46.0 0.0 0.0
14 0.0 -14.0 46.0 0.0 0.0
15 0.0 -16.0 44.0 0.0 0.0
16 0.0 -16.0 44.0 0.0 0.0
17 0.0 -14.0 46.0 0.0 0.0
18 0.0 -14.0 46.0 0.0 0.0
19 0.0 -14.0 46.0 0.0 0.0
20 0.0 -14.0 46.0 0.0 0.0
2025-03-04 21:48:24 Matching curves for turbine 001...
2025-03-04 21:48:25 T002 T006 T005 T003 T000
0 60.0 16.0 14.0 16.0 16.0
1 60.0 16.0 14.0 14.0 16.0
2 60.0 14.0 16.0 16.0 14.0
3 60.0 14.0 16.0 16.0 16.0
4 60.0 16.0 14.0 16.0 16.0
5 60.0 14.0 14.0 14.0 16.0
6 60.0 14.0 14.0 14.0 14.0
7 60.0 14.0 14.0 16.0 16.0
8 60.0 16.0 16.0 14.0 14.0
9 60.0 14.0 14.0 14.0 16.0
10 60.0 14.0 14.0 16.0 14.0
11 60.0 14.0 16.0 16.0 14.0
12 60.0 16.0 16.0 16.0 14.0
13 60.0 16.0 14.0 16.0 14.0
14 60.0 14.0 16.0 14.0 14.0
15 60.0 14.0 14.0 14.0 16.0
16 60.0 16.0 16.0 16.0 16.0
17 60.0 14.0 16.0 16.0 14.0
18 60.0 14.0 14.0 16.0 14.0
19 60.0 14.0 14.0 14.0 14.0
20 60.0 14.0 16.0 14.0 14.0
2025-03-04 21:48:25 Matching curves for turbine 002...
2025-03-04 21:48:25 T001 T003 T005 T000 T006
0 -60.0 -44.0 -46.0 -44.0 -44.0
1 -60.0 -46.0 -46.0 -44.0 -44.0
2 -60.0 -44.0 -44.0 -46.0 -46.0
3 -60.0 -44.0 -44.0 -44.0 -46.0
4 -60.0 -44.0 -46.0 -44.0 -44.0
5 -60.0 -46.0 -44.0 -44.0 -46.0
6 -60.0 -46.0 -46.0 -46.0 -46.0
7 -60.0 -44.0 -44.0 -44.0 -46.0
8 -60.0 -46.0 -44.0 -46.0 -44.0
9 -60.0 -46.0 -46.0 -44.0 -46.0
10 -60.0 -44.0 -46.0 -46.0 -46.0
11 -60.0 -44.0 -44.0 -46.0 -46.0
12 -60.0 -44.0 -44.0 -44.0 -44.0
13 -60.0 -44.0 -46.0 -46.0 -46.0
14 -60.0 -46.0 -44.0 -46.0 -46.0
15 -60.0 -46.0 -46.0 -44.0 -46.0
16 -60.0 -44.0 -44.0 -44.0 -44.0
17 -60.0 -44.0 -44.0 -46.0 -46.0
18 -60.0 -44.0 -46.0 -46.0 -46.0
19 -60.0 -46.0 -46.0 -46.0 -46.0
20 -60.0 -46.0 -44.0 -46.0 -46.0
2025-03-04 21:48:25 Matching curves for turbine 003...
2025-03-04 21:48:26 T005 T002 T001 T004 T006
0 0.0 44.0 -16.0 0.0 0.0
1 0.0 46.0 -14.0 0.0 0.0
2 0.0 44.0 -16.0 0.0 0.0
3 0.0 44.0 -16.0 0.0 0.0
4 0.0 44.0 -16.0 0.0 0.0
5 0.0 46.0 -14.0 0.0 0.0
6 0.0 46.0 -14.0 0.0 0.0
7 0.0 44.0 -16.0 0.0 0.0
8 0.0 46.0 -14.0 0.0 0.0
9 0.0 46.0 -14.0 0.0 0.0
10 0.0 44.0 -16.0 0.0 0.0
11 0.0 44.0 -16.0 0.0 0.0
12 0.0 44.0 -16.0 0.0 0.0
13 0.0 44.0 -16.0 0.0 0.0
14 0.0 46.0 -14.0 0.0 0.0
15 0.0 46.0 -14.0 0.0 0.0
16 0.0 44.0 -16.0 0.0 0.0
17 0.0 44.0 -16.0 0.0 0.0
18 0.0 44.0 -16.0 0.0 0.0
19 0.0 46.0 -14.0 0.0 0.0
20 0.0 46.0 -14.0 0.0 0.0
2025-03-04 21:48:26 Matching curves for turbine 004...
2025-03-04 21:48:26 T003 T002 T005 T001 T006
0 -0.0 46.0 0.0 -14.0 0.0
1 -0.0 44.0 0.0 -16.0 0.0
2 -0.0 44.0 0.0 -16.0 0.0
3 -0.0 46.0 0.0 -14.0 0.0
4 -0.0 46.0 0.0 -14.0 0.0
5 -0.0 44.0 0.0 -16.0 0.0
6 -0.0 44.0 0.0 -16.0 0.0
7 -0.0 44.0 0.0 -16.0 0.0
8 -0.0 44.0 0.0 -16.0 0.0
9 -0.0 46.0 0.0 -14.0 0.0
10 -0.0 44.0 0.0 -16.0 0.0
11 -0.0 46.0 0.0 -14.0 0.0
12 -0.0 46.0 0.0 -14.0 0.0
13 -0.0 44.0 0.0 -16.0 0.0
14 -0.0 46.0 0.0 -14.0 0.0
15 -0.0 44.0 0.0 -16.0 0.0
16 -0.0 46.0 0.0 -14.0 0.0
17 -0.0 44.0 0.0 -16.0 0.0
18 -0.0 46.0 0.0 -14.0 0.0
19 -0.0 44.0 0.0 -16.0 0.0
20 -0.0 44.0 0.0 -16.0 0.0
2025-03-04 21:48:26 Matching curves for turbine 005...
2025-03-04 21:48:26 T003 T001 T006 T002 T000
0 -0.0 -14.0 0.0 46.0 -0.0
1 -0.0 -14.0 0.0 46.0 -0.0
2 -0.0 -16.0 0.0 44.0 -0.0
3 -0.0 -16.0 0.0 44.0 -0.0
4 -0.0 -14.0 0.0 46.0 -0.0
5 -0.0 -14.0 0.0 44.0 -0.0
6 -0.0 -14.0 0.0 46.0 -0.0
7 -0.0 -14.0 0.0 44.0 -0.0
8 -0.0 -16.0 0.0 44.0 -0.0
9 -0.0 -14.0 0.0 46.0 -0.0
10 -0.0 -14.0 0.0 46.0 -0.0
11 -0.0 -16.0 0.0 44.0 -0.0
12 -0.0 -16.0 0.0 44.0 -0.0
13 -0.0 -14.0 0.0 46.0 -0.0
14 -0.0 -16.0 0.0 44.0 -0.0
15 -0.0 -14.0 0.0 46.0 -0.0
16 -0.0 -16.0 0.0 44.0 -0.0
17 -0.0 -16.0 0.0 44.0 -0.0
18 -0.0 -14.0 0.0 46.0 -0.0
19 -0.0 -14.0 0.0 46.0 -0.0
20 -0.0 -16.0 0.0 44.0 -0.0
2025-03-04 21:48:26 Matching curves for turbine 006...
2025-03-04 21:48:26 T001 T005 T000 T003 T002
0 -16.0 -0.0 -0.0 -0.0 44.0
1 -16.0 -0.0 -0.0 -0.0 44.0
2 -14.0 -0.0 -0.0 -0.0 46.0
3 -14.0 -0.0 -0.0 -0.0 46.0
4 -16.0 -0.0 -0.0 -0.0 44.0
5 -14.0 -0.0 -0.0 -0.0 46.0
6 -14.0 -0.0 -0.0 -0.0 46.0
7 -14.0 -0.0 -0.0 -0.0 46.0
8 -16.0 -0.0 -0.0 -0.0 44.0
9 -14.0 -0.0 -0.0 -0.0 46.0
10 -14.0 -0.0 -0.0 -0.0 46.0
11 -14.0 -0.0 -0.0 -0.0 46.0
12 -16.0 -0.0 -0.0 -0.0 44.0
13 -16.0 -0.0 -0.0 -0.0 46.0
14 -14.0 -0.0 -0.0 -0.0 46.0
15 -14.0 -0.0 -0.0 -0.0 46.0
16 -16.0 -0.0 -0.0 -0.0 44.0
17 -14.0 -0.0 -0.0 -0.0 46.0
18 -14.0 -0.0 -0.0 -0.0 46.0
19 -14.0 -0.0 -0.0 -0.0 46.0
20 -14.0 -0.0 -0.0 -0.0 46.0
2025-03-04 21:48:26 Turbine 000 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:26 Turbine 001 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:26 Turbine 002 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:26 Turbine 003 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:26 Turbine 004 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:26 Turbine 005 seems to have no jumps in its WD measurement calibration. [CLEAN]
2025-03-04 21:48:26 Turbine 006 seems to have no jumps in its WD measurement calibration. [CLEAN]
['clean', 'clean', 'clean', 'clean', 'clean', 'clean', 'clean']
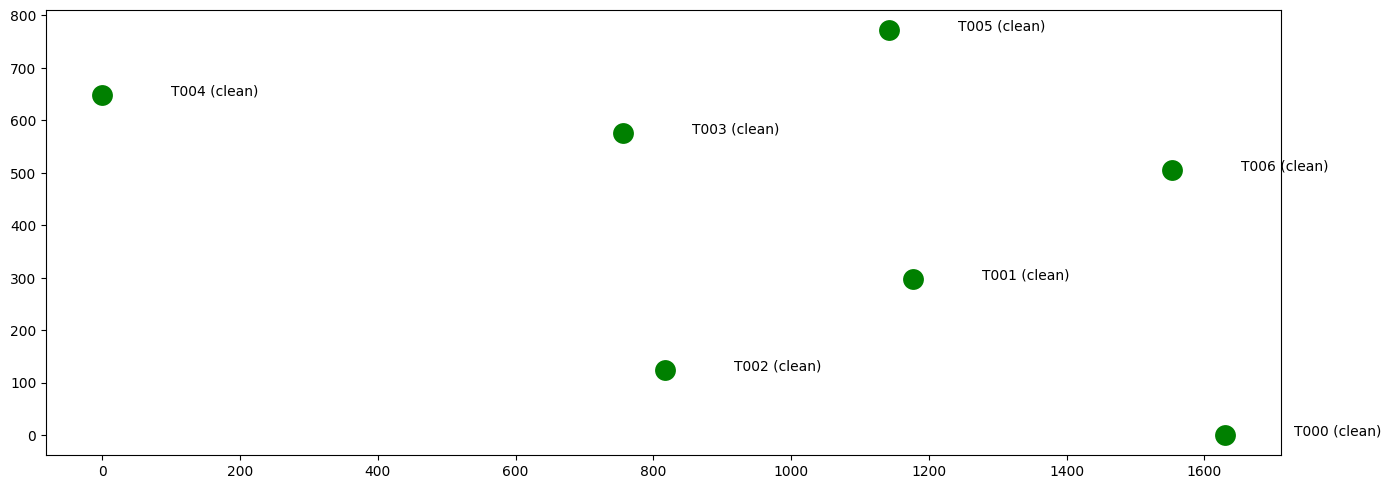
Remove steady offset from a single turbine#
By looking at the energy ratios and looking at the average offset between turbines' wind direction measurements, we can align every turbine that was flagged 'green' in the above plot with true north. Wind directions of turbines flagged red should not be used.
def get_bias_for_single_turbine(
df, fm, ti, opt_search_range=[-180.0, 180.0], plot=True, figure_save_path=None
):
print("Initializing wd bias estimator object for turbine %03d..." % ti)
# Copy variables and unlink them
df = df.copy() # Unlink from input
# Calculate which turbines are upstream for every wind direction
df_upstream = ftools.get_upstream_turbs_floris(fm, wd_step=2.0)
# We assign the total datasets "true" wind direction as equal to the wind
# direction of the turbine which we want to perform northing calibration
# on. In this case, turbine 'ti'.
df = dfm.set_wd_by_turbines(df, [ti])
# We define a function that calculates the freestream wind speed based
# on a dataframe that is inserted. It does this based on knowing which
# turbines are upstream for what wind directions, and then knowledge
# of what the wind direction is for every row in the dataframe. However,
# since the shift the "true" wind direction many times to estimate the
# northing bias, we cannot precalculate this. It changes with every
# northing bias guess. Hence, we must insert a function.
def _set_ws_fun(df):
return dfm.set_ws_by_upstream_turbines_in_radius(
df=df,
df_upstream=df_upstream,
turb_no=ti,
x_turbs=fm.layout_x,
y_turbs=fm.layout_y,
max_radius=5000.0,
include_itself=True,
)
# We similarly define a function that calculates the reference power. This
# is typically the power production of one or multiple upstream turbines.
# Here, we assume it is the average power production of all upstream
# turbines. Which turbines are upstream depends on the wind direction.
def _set_pow_ref_fun(df):
return dfm.set_pow_ref_by_upstream_turbines_in_radius(
df=df,
df_upstream=df_upstream,
turb_no=ti,
x_turbs=fm.layout_x,
y_turbs=fm.layout_y,
max_radius=5000.0,
include_itself=True,
)
# We now have the reference power productions specified, being equal to
# the mean power production of all turbines upstream. We also need to
# define a test power production, which should be waked at least part of
# the time so that we can match it with our FLORIS predictions. Here, we
# calculate the energy ratios for the 3 turbines closest to the turbine
# from which we take the wind direction measurement ('ti').
turbines_sorted_by_distance = ftools.get_turbs_in_radius(
x_turbs=fm.layout_x,
y_turbs=fm.layout_y,
turb_no=ti,
max_radius=1.0e9,
include_itself=False,
sort_by_distance=True,
)
test_turbines = turbines_sorted_by_distance[0:3]
# Now, we have all information set up and we can initialize the northing
# bias estimation class.
fsc = best.bias_estimation(
df=df,
df_fm_approx=df_fm_approx,
test_turbines_subset=test_turbines,
df_ws_mapping_func=_set_ws_fun,
df_pow_ref_mapping_func=_set_pow_ref_fun,
)
# We can save the energy ratio curves for every iteration in the
# optimization process. This is useful for debugging. However, it also
# significantly slows down the estimation process. We disable it by
# default by assigning it 'None'.
plot_iter_path = None # Disabled, useful for debugging but slow
# plot_iter_path = os.path.join(out_path, "opt_iters_ti%03d" % ti)
# Now estimate the wind direction bias while catching warning messages
# that do not really inform but do pollute the console.
with wn.catch_warnings():
wn.filterwarnings(action="ignore", message="All-NaN slice encountered")
# Estimate bias for the entire time range, from start to end of
# dataframe, for wind speeds in region II of turbine operation, with
# in steps of 3.0 deg (wd) and 5.0 m/s (ws). We search over the entire
# range from -180.0 deg to +180.0 deg, in steps of 5.0 deg. This has
# appeared to be a good stepsize empirically.
wd_bias, _ = fsc.estimate_wd_bias(
time_mask=None, # For entire dataset
ws_mask=(6.0, 10.0),
er_wd_step=3.0,
er_ws_step=5.0,
er_wd_bin_width=3.0,
er_N_btstrp=1,
opt_search_brute_dx=5.0,
opt_search_range=opt_search_range,
plot_iter_path=plot_iter_path,
)
wd_bias = float(wd_bias[0]) # Convert to float
# Print progress to console
print("Turbine {}. estimated bias = {} deg.".format(ti, wd_bias))
if plot:
# Produce and save calibrated/corrected energy ratio figures
fsc.plot_energy_ratios(show_uncorrected_data=True, save_path=figure_save_path)
if figure_save_path is not None:
print("Calibrated energy ratio figures saved to {:s}.".format(figure_save_path))
# Finally, return the estimated wind direction bias
return wd_bias
# We will calibrate the turbine nacelle heading for the first 'clean' turbine
first_clean_turbid = np.where([c == "clean" for c in turb_wd_consistency])[0][0]
# Calculate optimal bias for the first clean turbine, covering all possibilities
# (from -180 deg to +180 deg offset)
wd_bias = get_bias_for_single_turbine(
df=df_scada_homogenized,
fm=fm,
ti=first_clean_turbid,
opt_search_range=(-180.0, 180.0),
plot=True,
)
print("WD bias for first clean turbine: {:.3f} deg".format(wd_bias))
# Now calculate the northing-bias-corrected wind direction for this
# turbine and call it our reference
wd_ref = wrap_360(df_scada_homogenized["wd_{:03d}".format(first_clean_turbid)] - wd_bias)
2025-03-04 21:48:27 Initializing a bias_estimation() object...
2025-03-04 21:48:27 Estimating the wind direction bias
2025-03-04 21:48:27 Initializing energy ratio inputs.
2025-03-04 21:48:27 Constructing energy table for wd_bias of -180.00 deg.
Initializing wd bias estimator object for turbine 000...
2025-03-04 21:48:27 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:27 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:27 minimum/maximum value in df: (7.798, 8.248)
2025-03-04 21:48:27 minimum/maximum value in df: (7.798, 8.248)
2025-03-04 21:48:27 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:27 Determining energy ratios for test turbine = 006. WD bias: -180.000 deg.
2025-03-04 21:48:27 Determining energy ratios for test turbine = 001. WD bias: -180.000 deg.
2025-03-04 21:48:27 Determining energy ratios for test turbine = 002. WD bias: -180.000 deg.
2025-03-04 21:48:27 Initializing energy ratio inputs.
2025-03-04 21:48:27 Constructing energy table for wd_bias of -175.00 deg.
2025-03-04 21:48:27 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:27 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:27 minimum/maximum value in df: (7.709, 8.248)
2025-03-04 21:48:27 minimum/maximum value in df: (7.709, 8.248)
2025-03-04 21:48:27 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:27 Determining energy ratios for test turbine = 006. WD bias: -175.000 deg.
2025-03-04 21:48:27 Determining energy ratios for test turbine = 001. WD bias: -175.000 deg.
2025-03-04 21:48:27 Determining energy ratios for test turbine = 002. WD bias: -175.000 deg.
2025-03-04 21:48:27 Initializing energy ratio inputs.
2025-03-04 21:48:27 Constructing energy table for wd_bias of -170.00 deg.
2025-03-04 21:48:27 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:28 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:28 minimum/maximum value in df: (7.709, 8.243)
2025-03-04 21:48:28 minimum/maximum value in df: (7.709, 8.243)
2025-03-04 21:48:28 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:28 Determining energy ratios for test turbine = 006. WD bias: -170.000 deg.
2025-03-04 21:48:28 Determining energy ratios for test turbine = 001. WD bias: -170.000 deg.
2025-03-04 21:48:28 Determining energy ratios for test turbine = 002. WD bias: -170.000 deg.
2025-03-04 21:48:28 Initializing energy ratio inputs.
2025-03-04 21:48:28 Constructing energy table for wd_bias of -165.00 deg.
2025-03-04 21:48:28 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:28 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:28 minimum/maximum value in df: (7.799, 8.243)
2025-03-04 21:48:28 minimum/maximum value in df: (7.799, 8.243)
2025-03-04 21:48:28 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:28 Determining energy ratios for test turbine = 006. WD bias: -165.000 deg.
2025-03-04 21:48:28 Determining energy ratios for test turbine = 001. WD bias: -165.000 deg.
2025-03-04 21:48:28 Determining energy ratios for test turbine = 002. WD bias: -165.000 deg.
2025-03-04 21:48:28 Initializing energy ratio inputs.
2025-03-04 21:48:28 Constructing energy table for wd_bias of -160.00 deg.
2025-03-04 21:48:28 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:28 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:28 minimum/maximum value in df: (7.799, 8.230)
2025-03-04 21:48:28 minimum/maximum value in df: (7.799, 8.230)
2025-03-04 21:48:28 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:28 Determining energy ratios for test turbine = 006. WD bias: -160.000 deg.
2025-03-04 21:48:28 Determining energy ratios for test turbine = 001. WD bias: -160.000 deg.
2025-03-04 21:48:28 Determining energy ratios for test turbine = 002. WD bias: -160.000 deg.
2025-03-04 21:48:28 Initializing energy ratio inputs.
2025-03-04 21:48:28 Constructing energy table for wd_bias of -155.00 deg.
2025-03-04 21:48:29 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:29 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:29 minimum/maximum value in df: (7.788, 8.230)
2025-03-04 21:48:29 minimum/maximum value in df: (7.788, 8.230)
2025-03-04 21:48:29 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:29 Determining energy ratios for test turbine = 006. WD bias: -155.000 deg.
2025-03-04 21:48:29 Determining energy ratios for test turbine = 001. WD bias: -155.000 deg.
2025-03-04 21:48:29 Determining energy ratios for test turbine = 002. WD bias: -155.000 deg.
2025-03-04 21:48:29 Initializing energy ratio inputs.
2025-03-04 21:48:29 Constructing energy table for wd_bias of -150.00 deg.
2025-03-04 21:48:29 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:29 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:29 minimum/maximum value in df: (7.788, 8.227)
2025-03-04 21:48:29 minimum/maximum value in df: (7.788, 8.227)
2025-03-04 21:48:29 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:29 Determining energy ratios for test turbine = 006. WD bias: -150.000 deg.
2025-03-04 21:48:29 Determining energy ratios for test turbine = 001. WD bias: -150.000 deg.
2025-03-04 21:48:29 Determining energy ratios for test turbine = 002. WD bias: -150.000 deg.
2025-03-04 21:48:29 Initializing energy ratio inputs.
2025-03-04 21:48:29 Constructing energy table for wd_bias of -145.00 deg.
2025-03-04 21:48:29 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:29 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:29 minimum/maximum value in df: (7.794, 8.227)
2025-03-04 21:48:29 minimum/maximum value in df: (7.794, 8.227)
2025-03-04 21:48:29 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:29 Determining energy ratios for test turbine = 006. WD bias: -145.000 deg.
2025-03-04 21:48:29 Determining energy ratios for test turbine = 001. WD bias: -145.000 deg.
2025-03-04 21:48:29 Determining energy ratios for test turbine = 002. WD bias: -145.000 deg.
2025-03-04 21:48:30 Initializing energy ratio inputs.
2025-03-04 21:48:30 Constructing energy table for wd_bias of -140.00 deg.
2025-03-04 21:48:30 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:30 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:30 minimum/maximum value in df: (7.794, 8.227)
2025-03-04 21:48:30 minimum/maximum value in df: (7.794, 8.227)
2025-03-04 21:48:30 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:30 Determining energy ratios for test turbine = 006. WD bias: -140.000 deg.
2025-03-04 21:48:30 Determining energy ratios for test turbine = 001. WD bias: -140.000 deg.
2025-03-04 21:48:30 Determining energy ratios for test turbine = 002. WD bias: -140.000 deg.
2025-03-04 21:48:30 Initializing energy ratio inputs.
2025-03-04 21:48:30 Constructing energy table for wd_bias of -135.00 deg.
2025-03-04 21:48:30 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:30 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:30 minimum/maximum value in df: (7.811, 8.227)
2025-03-04 21:48:30 minimum/maximum value in df: (7.811, 8.227)
2025-03-04 21:48:30 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:30 Determining energy ratios for test turbine = 006. WD bias: -135.000 deg.
2025-03-04 21:48:30 Determining energy ratios for test turbine = 001. WD bias: -135.000 deg.
2025-03-04 21:48:30 Determining energy ratios for test turbine = 002. WD bias: -135.000 deg.
2025-03-04 21:48:30 Initializing energy ratio inputs.
2025-03-04 21:48:30 Constructing energy table for wd_bias of -130.00 deg.
2025-03-04 21:48:30 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:30 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:30 minimum/maximum value in df: (7.811, 8.219)
2025-03-04 21:48:30 minimum/maximum value in df: (7.811, 8.219)
2025-03-04 21:48:30 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:30 Determining energy ratios for test turbine = 006. WD bias: -130.000 deg.
2025-03-04 21:48:30 Determining energy ratios for test turbine = 001. WD bias: -130.000 deg.
2025-03-04 21:48:31 Determining energy ratios for test turbine = 002. WD bias: -130.000 deg.
2025-03-04 21:48:31 Initializing energy ratio inputs.
2025-03-04 21:48:31 Constructing energy table for wd_bias of -125.00 deg.
2025-03-04 21:48:31 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:31 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:31 minimum/maximum value in df: (7.795, 8.244)
2025-03-04 21:48:31 minimum/maximum value in df: (7.795, 8.244)
2025-03-04 21:48:31 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:31 Determining energy ratios for test turbine = 006. WD bias: -125.000 deg.
2025-03-04 21:48:31 Determining energy ratios for test turbine = 001. WD bias: -125.000 deg.
2025-03-04 21:48:31 Determining energy ratios for test turbine = 002. WD bias: -125.000 deg.
2025-03-04 21:48:31 Initializing energy ratio inputs.
2025-03-04 21:48:31 Constructing energy table for wd_bias of -120.00 deg.
2025-03-04 21:48:31 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:31 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:31 minimum/maximum value in df: (7.795, 8.244)
2025-03-04 21:48:31 minimum/maximum value in df: (7.795, 8.244)
2025-03-04 21:48:31 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:31 Determining energy ratios for test turbine = 006. WD bias: -120.000 deg.
2025-03-04 21:48:31 Determining energy ratios for test turbine = 001. WD bias: -120.000 deg.
2025-03-04 21:48:31 Determining energy ratios for test turbine = 002. WD bias: -120.000 deg.
2025-03-04 21:48:31 Initializing energy ratio inputs.
2025-03-04 21:48:31 Constructing energy table for wd_bias of -115.00 deg.
2025-03-04 21:48:31 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:31 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:31 minimum/maximum value in df: (7.795, 8.192)
2025-03-04 21:48:31 minimum/maximum value in df: (7.795, 8.192)
2025-03-04 21:48:31 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:31 Determining energy ratios for test turbine = 006. WD bias: -115.000 deg.
2025-03-04 21:48:32 Determining energy ratios for test turbine = 001. WD bias: -115.000 deg.
2025-03-04 21:48:32 Determining energy ratios for test turbine = 002. WD bias: -115.000 deg.
2025-03-04 21:48:32 Initializing energy ratio inputs.
2025-03-04 21:48:32 Constructing energy table for wd_bias of -110.00 deg.
2025-03-04 21:48:32 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:32 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:32 minimum/maximum value in df: (7.795, 8.198)
2025-03-04 21:48:32 minimum/maximum value in df: (7.795, 8.198)
2025-03-04 21:48:32 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:32 Determining energy ratios for test turbine = 006. WD bias: -110.000 deg.
2025-03-04 21:48:32 Determining energy ratios for test turbine = 001. WD bias: -110.000 deg.
2025-03-04 21:48:32 Determining energy ratios for test turbine = 002. WD bias: -110.000 deg.
2025-03-04 21:48:32 Initializing energy ratio inputs.
2025-03-04 21:48:32 Constructing energy table for wd_bias of -105.00 deg.
2025-03-04 21:48:32 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:32 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:32 minimum/maximum value in df: (7.803, 8.198)
2025-03-04 21:48:32 minimum/maximum value in df: (7.803, 8.198)
2025-03-04 21:48:32 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:32 Determining energy ratios for test turbine = 006. WD bias: -105.000 deg.
2025-03-04 21:48:32 Determining energy ratios for test turbine = 001. WD bias: -105.000 deg.
2025-03-04 21:48:32 Determining energy ratios for test turbine = 002. WD bias: -105.000 deg.
2025-03-04 21:48:32 Initializing energy ratio inputs.
2025-03-04 21:48:32 Constructing energy table for wd_bias of -100.00 deg.
2025-03-04 21:48:32 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:32 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:32 minimum/maximum value in df: (7.802, 8.219)
2025-03-04 21:48:32 minimum/maximum value in df: (7.802, 8.219)
2025-03-04 21:48:32 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:33 Determining energy ratios for test turbine = 006. WD bias: -100.000 deg.
2025-03-04 21:48:33 Determining energy ratios for test turbine = 001. WD bias: -100.000 deg.
2025-03-04 21:48:33 Determining energy ratios for test turbine = 002. WD bias: -100.000 deg.
2025-03-04 21:48:33 Initializing energy ratio inputs.
2025-03-04 21:48:33 Constructing energy table for wd_bias of -95.00 deg.
2025-03-04 21:48:33 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:33 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:33 minimum/maximum value in df: (7.795, 8.219)
2025-03-04 21:48:33 minimum/maximum value in df: (7.795, 8.219)
2025-03-04 21:48:33 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:33 Determining energy ratios for test turbine = 006. WD bias: -95.000 deg.
2025-03-04 21:48:33 Determining energy ratios for test turbine = 001. WD bias: -95.000 deg.
2025-03-04 21:48:33 Determining energy ratios for test turbine = 002. WD bias: -95.000 deg.
2025-03-04 21:48:33 Initializing energy ratio inputs.
2025-03-04 21:48:33 Constructing energy table for wd_bias of -90.00 deg.
2025-03-04 21:48:33 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:33 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:33 minimum/maximum value in df: (7.795, 8.249)
2025-03-04 21:48:33 minimum/maximum value in df: (7.795, 8.249)
2025-03-04 21:48:33 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:33 Determining energy ratios for test turbine = 006. WD bias: -90.000 deg.
2025-03-04 21:48:33 Determining energy ratios for test turbine = 001. WD bias: -90.000 deg.
2025-03-04 21:48:33 Determining energy ratios for test turbine = 002. WD bias: -90.000 deg.
2025-03-04 21:48:33 Initializing energy ratio inputs.
2025-03-04 21:48:33 Constructing energy table for wd_bias of -85.00 deg.
2025-03-04 21:48:33 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:33 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:33 minimum/maximum value in df: (7.795, 8.249)
2025-03-04 21:48:33 minimum/maximum value in df: (7.795, 8.249)
2025-03-04 21:48:33 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:34 Determining energy ratios for test turbine = 006. WD bias: -85.000 deg.
2025-03-04 21:48:34 Determining energy ratios for test turbine = 001. WD bias: -85.000 deg.
2025-03-04 21:48:34 Determining energy ratios for test turbine = 002. WD bias: -85.000 deg.
2025-03-04 21:48:34 Initializing energy ratio inputs.
2025-03-04 21:48:34 Constructing energy table for wd_bias of -80.00 deg.
2025-03-04 21:48:34 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:34 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:34 minimum/maximum value in df: (7.725, 8.227)
2025-03-04 21:48:34 minimum/maximum value in df: (7.725, 8.227)
2025-03-04 21:48:34 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:34 Determining energy ratios for test turbine = 006. WD bias: -80.000 deg.
2025-03-04 21:48:34 Determining energy ratios for test turbine = 001. WD bias: -80.000 deg.
2025-03-04 21:48:34 Determining energy ratios for test turbine = 002. WD bias: -80.000 deg.
2025-03-04 21:48:34 Initializing energy ratio inputs.
2025-03-04 21:48:34 Constructing energy table for wd_bias of -75.00 deg.
2025-03-04 21:48:34 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:34 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:34 minimum/maximum value in df: (7.725, 8.227)
2025-03-04 21:48:34 minimum/maximum value in df: (7.725, 8.227)
2025-03-04 21:48:34 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:34 Determining energy ratios for test turbine = 006. WD bias: -75.000 deg.
2025-03-04 21:48:34 Determining energy ratios for test turbine = 001. WD bias: -75.000 deg.
2025-03-04 21:48:34 Determining energy ratios for test turbine = 002. WD bias: -75.000 deg.
2025-03-04 21:48:34 Initializing energy ratio inputs.
2025-03-04 21:48:34 Constructing energy table for wd_bias of -70.00 deg.
2025-03-04 21:48:34 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:34 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:34 minimum/maximum value in df: (7.810, 8.227)
2025-03-04 21:48:34 minimum/maximum value in df: (7.810, 8.227)
2025-03-04 21:48:34 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:35 Determining energy ratios for test turbine = 006. WD bias: -70.000 deg.
2025-03-04 21:48:35 Determining energy ratios for test turbine = 001. WD bias: -70.000 deg.
2025-03-04 21:48:35 Determining energy ratios for test turbine = 002. WD bias: -70.000 deg.
2025-03-04 21:48:35 Initializing energy ratio inputs.
2025-03-04 21:48:35 Constructing energy table for wd_bias of -65.00 deg.
2025-03-04 21:48:35 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:35 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:35 minimum/maximum value in df: (7.773, 8.234)
2025-03-04 21:48:35 minimum/maximum value in df: (7.773, 8.234)
2025-03-04 21:48:35 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:35 Determining energy ratios for test turbine = 006. WD bias: -65.000 deg.
2025-03-04 21:48:35 Determining energy ratios for test turbine = 001. WD bias: -65.000 deg.
2025-03-04 21:48:35 Determining energy ratios for test turbine = 002. WD bias: -65.000 deg.
2025-03-04 21:48:35 Initializing energy ratio inputs.
2025-03-04 21:48:35 Constructing energy table for wd_bias of -60.00 deg.
2025-03-04 21:48:35 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:35 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:35 minimum/maximum value in df: (7.773, 8.234)
2025-03-04 21:48:35 minimum/maximum value in df: (7.773, 8.234)
2025-03-04 21:48:35 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:35 Determining energy ratios for test turbine = 006. WD bias: -60.000 deg.
2025-03-04 21:48:35 Determining energy ratios for test turbine = 001. WD bias: -60.000 deg.
2025-03-04 21:48:35 Determining energy ratios for test turbine = 002. WD bias: -60.000 deg.
2025-03-04 21:48:35 Initializing energy ratio inputs.
2025-03-04 21:48:35 Constructing energy table for wd_bias of -55.00 deg.
2025-03-04 21:48:35 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:35 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:35 minimum/maximum value in df: (7.810, 8.234)
2025-03-04 21:48:35 minimum/maximum value in df: (7.810, 8.234)
2025-03-04 21:48:35 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:36 Determining energy ratios for test turbine = 006. WD bias: -55.000 deg.
2025-03-04 21:48:36 Determining energy ratios for test turbine = 001. WD bias: -55.000 deg.
2025-03-04 21:48:36 Determining energy ratios for test turbine = 002. WD bias: -55.000 deg.
2025-03-04 21:48:36 Initializing energy ratio inputs.
2025-03-04 21:48:36 Constructing energy table for wd_bias of -50.00 deg.
2025-03-04 21:48:36 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:36 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:36 minimum/maximum value in df: (7.798, 8.234)
2025-03-04 21:48:36 minimum/maximum value in df: (7.798, 8.234)
2025-03-04 21:48:36 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:36 Determining energy ratios for test turbine = 006. WD bias: -50.000 deg.
2025-03-04 21:48:36 Determining energy ratios for test turbine = 001. WD bias: -50.000 deg.
2025-03-04 21:48:36 Determining energy ratios for test turbine = 002. WD bias: -50.000 deg.
2025-03-04 21:48:36 Initializing energy ratio inputs.
2025-03-04 21:48:36 Constructing energy table for wd_bias of -45.00 deg.
2025-03-04 21:48:36 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:36 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:36 minimum/maximum value in df: (7.783, 8.222)
2025-03-04 21:48:36 minimum/maximum value in df: (7.783, 8.222)
2025-03-04 21:48:36 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:36 Determining energy ratios for test turbine = 006. WD bias: -45.000 deg.
2025-03-04 21:48:36 Determining energy ratios for test turbine = 001. WD bias: -45.000 deg.
2025-03-04 21:48:36 Determining energy ratios for test turbine = 002. WD bias: -45.000 deg.
2025-03-04 21:48:36 Initializing energy ratio inputs.
2025-03-04 21:48:36 Constructing energy table for wd_bias of -40.00 deg.
2025-03-04 21:48:37 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:37 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:37 minimum/maximum value in df: (7.783, 8.235)
2025-03-04 21:48:37 minimum/maximum value in df: (7.783, 8.235)
2025-03-04 21:48:37 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:37 Determining energy ratios for test turbine = 006. WD bias: -40.000 deg.
2025-03-04 21:48:37 Determining energy ratios for test turbine = 001. WD bias: -40.000 deg.
2025-03-04 21:48:37 Determining energy ratios for test turbine = 002. WD bias: -40.000 deg.
2025-03-04 21:48:37 Initializing energy ratio inputs.
2025-03-04 21:48:37 Constructing energy table for wd_bias of -35.00 deg.
2025-03-04 21:48:37 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:37 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:37 minimum/maximum value in df: (7.783, 8.235)
2025-03-04 21:48:37 minimum/maximum value in df: (7.783, 8.235)
2025-03-04 21:48:37 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:37 Determining energy ratios for test turbine = 006. WD bias: -35.000 deg.
2025-03-04 21:48:37 Determining energy ratios for test turbine = 001. WD bias: -35.000 deg.
2025-03-04 21:48:37 Determining energy ratios for test turbine = 002. WD bias: -35.000 deg.
2025-03-04 21:48:37 Initializing energy ratio inputs.
2025-03-04 21:48:37 Constructing energy table for wd_bias of -30.00 deg.
2025-03-04 21:48:37 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:37 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:37 minimum/maximum value in df: (7.766, 8.240)
2025-03-04 21:48:37 minimum/maximum value in df: (7.766, 8.240)
2025-03-04 21:48:37 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:37 Determining energy ratios for test turbine = 006. WD bias: -30.000 deg.
2025-03-04 21:48:37 Determining energy ratios for test turbine = 001. WD bias: -30.000 deg.
2025-03-04 21:48:37 Determining energy ratios for test turbine = 002. WD bias: -30.000 deg.
2025-03-04 21:48:37 Initializing energy ratio inputs.
2025-03-04 21:48:37 Constructing energy table for wd_bias of -25.00 deg.
2025-03-04 21:48:38 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:38 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:38 minimum/maximum value in df: (7.766, 8.253)
2025-03-04 21:48:38 minimum/maximum value in df: (7.766, 8.253)
2025-03-04 21:48:38 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:38 Determining energy ratios for test turbine = 006. WD bias: -25.000 deg.
2025-03-04 21:48:38 Determining energy ratios for test turbine = 001. WD bias: -25.000 deg.
2025-03-04 21:48:38 Determining energy ratios for test turbine = 002. WD bias: -25.000 deg.
2025-03-04 21:48:38 Initializing energy ratio inputs.
2025-03-04 21:48:38 Constructing energy table for wd_bias of -20.00 deg.
2025-03-04 21:48:38 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:38 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:38 minimum/maximum value in df: (7.787, 8.253)
2025-03-04 21:48:38 minimum/maximum value in df: (7.787, 8.253)
2025-03-04 21:48:38 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:38 Determining energy ratios for test turbine = 006. WD bias: -20.000 deg.
2025-03-04 21:48:38 Determining energy ratios for test turbine = 001. WD bias: -20.000 deg.
2025-03-04 21:48:38 Determining energy ratios for test turbine = 002. WD bias: -20.000 deg.
2025-03-04 21:48:38 Initializing energy ratio inputs.
2025-03-04 21:48:38 Constructing energy table for wd_bias of -15.00 deg.
2025-03-04 21:48:38 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:38 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:38 minimum/maximum value in df: (7.776, 8.253)
2025-03-04 21:48:38 minimum/maximum value in df: (7.776, 8.253)
2025-03-04 21:48:38 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:38 Determining energy ratios for test turbine = 006. WD bias: -15.000 deg.
2025-03-04 21:48:38 Determining energy ratios for test turbine = 001. WD bias: -15.000 deg.
2025-03-04 21:48:38 Determining energy ratios for test turbine = 002. WD bias: -15.000 deg.
2025-03-04 21:48:39 Initializing energy ratio inputs.
2025-03-04 21:48:39 Constructing energy table for wd_bias of -10.00 deg.
2025-03-04 21:48:39 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:39 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:39 minimum/maximum value in df: (7.776, 8.253)
2025-03-04 21:48:39 minimum/maximum value in df: (7.776, 8.253)
2025-03-04 21:48:39 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:39 Determining energy ratios for test turbine = 006. WD bias: -10.000 deg.
2025-03-04 21:48:39 Determining energy ratios for test turbine = 001. WD bias: -10.000 deg.
2025-03-04 21:48:39 Determining energy ratios for test turbine = 002. WD bias: -10.000 deg.
2025-03-04 21:48:39 Initializing energy ratio inputs.
2025-03-04 21:48:39 Constructing energy table for wd_bias of -5.00 deg.
2025-03-04 21:48:39 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:39 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:39 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:48:39 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:48:39 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:39 Determining energy ratios for test turbine = 006. WD bias: -5.000 deg.
2025-03-04 21:48:39 Determining energy ratios for test turbine = 001. WD bias: -5.000 deg.
2025-03-04 21:48:39 Determining energy ratios for test turbine = 002. WD bias: -5.000 deg.
2025-03-04 21:48:39 Initializing energy ratio inputs.
2025-03-04 21:48:39 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:39 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:39 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:39 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:39 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:39 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:39 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:40 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:40 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:48:40 Initializing energy ratio inputs.
2025-03-04 21:48:40 Constructing energy table for wd_bias of 5.00 deg.
2025-03-04 21:48:40 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:40 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:40 minimum/maximum value in df: (7.789, 8.231)
2025-03-04 21:48:40 minimum/maximum value in df: (7.789, 8.231)
2025-03-04 21:48:40 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:40 Determining energy ratios for test turbine = 006. WD bias: 5.000 deg.
2025-03-04 21:48:40 Determining energy ratios for test turbine = 001. WD bias: 5.000 deg.
2025-03-04 21:48:40 Determining energy ratios for test turbine = 002. WD bias: 5.000 deg.
2025-03-04 21:48:40 Initializing energy ratio inputs.
2025-03-04 21:48:40 Constructing energy table for wd_bias of 10.00 deg.
2025-03-04 21:48:40 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:40 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:40 minimum/maximum value in df: (7.803, 8.222)
2025-03-04 21:48:40 minimum/maximum value in df: (7.803, 8.222)
2025-03-04 21:48:40 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:40 Determining energy ratios for test turbine = 006. WD bias: 10.000 deg.
2025-03-04 21:48:40 Determining energy ratios for test turbine = 001. WD bias: 10.000 deg.
2025-03-04 21:48:40 Determining energy ratios for test turbine = 002. WD bias: 10.000 deg.
2025-03-04 21:48:40 Initializing energy ratio inputs.
2025-03-04 21:48:40 Constructing energy table for wd_bias of 15.00 deg.
2025-03-04 21:48:40 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:40 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:40 minimum/maximum value in df: (7.803, 8.222)
2025-03-04 21:48:40 minimum/maximum value in df: (7.803, 8.222)
2025-03-04 21:48:40 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:41 Determining energy ratios for test turbine = 006. WD bias: 15.000 deg.
2025-03-04 21:48:41 Determining energy ratios for test turbine = 001. WD bias: 15.000 deg.
2025-03-04 21:48:41 Determining energy ratios for test turbine = 002. WD bias: 15.000 deg.
2025-03-04 21:48:41 Initializing energy ratio inputs.
2025-03-04 21:48:41 Constructing energy table for wd_bias of 20.00 deg.
2025-03-04 21:48:41 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:41 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:41 minimum/maximum value in df: (7.794, 8.222)
2025-03-04 21:48:41 minimum/maximum value in df: (7.794, 8.222)
2025-03-04 21:48:41 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:41 Determining energy ratios for test turbine = 006. WD bias: 20.000 deg.
2025-03-04 21:48:41 Determining energy ratios for test turbine = 001. WD bias: 20.000 deg.
2025-03-04 21:48:41 Determining energy ratios for test turbine = 002. WD bias: 20.000 deg.
2025-03-04 21:48:41 Initializing energy ratio inputs.
2025-03-04 21:48:41 Constructing energy table for wd_bias of 25.00 deg.
2025-03-04 21:48:41 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:41 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:41 minimum/maximum value in df: (7.794, 8.222)
2025-03-04 21:48:41 minimum/maximum value in df: (7.794, 8.222)
2025-03-04 21:48:41 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:41 Determining energy ratios for test turbine = 006. WD bias: 25.000 deg.
2025-03-04 21:48:41 Determining energy ratios for test turbine = 001. WD bias: 25.000 deg.
2025-03-04 21:48:41 Determining energy ratios for test turbine = 002. WD bias: 25.000 deg.
2025-03-04 21:48:41 Initializing energy ratio inputs.
2025-03-04 21:48:41 Constructing energy table for wd_bias of 30.00 deg.
2025-03-04 21:48:42 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:42 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:42 minimum/maximum value in df: (7.794, 8.193)
2025-03-04 21:48:42 minimum/maximum value in df: (7.794, 8.193)
2025-03-04 21:48:42 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:42 Determining energy ratios for test turbine = 006. WD bias: 30.000 deg.
2025-03-04 21:48:42 Determining energy ratios for test turbine = 001. WD bias: 30.000 deg.
2025-03-04 21:48:42 Determining energy ratios for test turbine = 002. WD bias: 30.000 deg.
2025-03-04 21:48:42 Initializing energy ratio inputs.
2025-03-04 21:48:42 Constructing energy table for wd_bias of 35.00 deg.
2025-03-04 21:48:42 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:42 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:42 minimum/maximum value in df: (7.794, 8.230)
2025-03-04 21:48:42 minimum/maximum value in df: (7.794, 8.230)
2025-03-04 21:48:42 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:42 Determining energy ratios for test turbine = 006. WD bias: 35.000 deg.
2025-03-04 21:48:42 Determining energy ratios for test turbine = 001. WD bias: 35.000 deg.
2025-03-04 21:48:42 Determining energy ratios for test turbine = 002. WD bias: 35.000 deg.
2025-03-04 21:48:42 Initializing energy ratio inputs.
2025-03-04 21:48:42 Constructing energy table for wd_bias of 40.00 deg.
2025-03-04 21:48:42 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:42 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:42 minimum/maximum value in df: (7.796, 8.239)
2025-03-04 21:48:42 minimum/maximum value in df: (7.796, 8.239)
2025-03-04 21:48:42 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:42 Determining energy ratios for test turbine = 006. WD bias: 40.000 deg.
2025-03-04 21:48:42 Determining energy ratios for test turbine = 001. WD bias: 40.000 deg.
2025-03-04 21:48:42 Determining energy ratios for test turbine = 002. WD bias: 40.000 deg.
2025-03-04 21:48:42 Initializing energy ratio inputs.
2025-03-04 21:48:42 Constructing energy table for wd_bias of 45.00 deg.
2025-03-04 21:48:43 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:43 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:43 minimum/maximum value in df: (7.802, 8.239)
2025-03-04 21:48:43 minimum/maximum value in df: (7.802, 8.239)
2025-03-04 21:48:43 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:43 Determining energy ratios for test turbine = 006. WD bias: 45.000 deg.
2025-03-04 21:48:43 Determining energy ratios for test turbine = 001. WD bias: 45.000 deg.
2025-03-04 21:48:43 Determining energy ratios for test turbine = 002. WD bias: 45.000 deg.
2025-03-04 21:48:43 Initializing energy ratio inputs.
2025-03-04 21:48:43 Constructing energy table for wd_bias of 50.00 deg.
2025-03-04 21:48:43 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:43 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:43 minimum/maximum value in df: (7.802, 8.239)
2025-03-04 21:48:43 minimum/maximum value in df: (7.802, 8.239)
2025-03-04 21:48:43 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:43 Determining energy ratios for test turbine = 006. WD bias: 50.000 deg.
2025-03-04 21:48:43 Determining energy ratios for test turbine = 001. WD bias: 50.000 deg.
2025-03-04 21:48:43 Determining energy ratios for test turbine = 002. WD bias: 50.000 deg.
2025-03-04 21:48:43 Initializing energy ratio inputs.
2025-03-04 21:48:43 Constructing energy table for wd_bias of 55.00 deg.
2025-03-04 21:48:43 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:43 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:43 minimum/maximum value in df: (7.795, 8.239)
2025-03-04 21:48:43 minimum/maximum value in df: (7.795, 8.239)
2025-03-04 21:48:43 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:43 Determining energy ratios for test turbine = 006. WD bias: 55.000 deg.
2025-03-04 21:48:43 Determining energy ratios for test turbine = 001. WD bias: 55.000 deg.
2025-03-04 21:48:43 Determining energy ratios for test turbine = 002. WD bias: 55.000 deg.
2025-03-04 21:48:44 Initializing energy ratio inputs.
2025-03-04 21:48:44 Constructing energy table for wd_bias of 60.00 deg.
2025-03-04 21:48:44 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:44 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:44 minimum/maximum value in df: (7.795, 8.220)
2025-03-04 21:48:44 minimum/maximum value in df: (7.795, 8.220)
2025-03-04 21:48:44 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:44 Determining energy ratios for test turbine = 006. WD bias: 60.000 deg.
2025-03-04 21:48:44 Determining energy ratios for test turbine = 001. WD bias: 60.000 deg.
2025-03-04 21:48:44 Determining energy ratios for test turbine = 002. WD bias: 60.000 deg.
2025-03-04 21:48:44 Initializing energy ratio inputs.
2025-03-04 21:48:44 Constructing energy table for wd_bias of 65.00 deg.
2025-03-04 21:48:44 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:44 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:44 minimum/maximum value in df: (7.795, 8.329)
2025-03-04 21:48:44 minimum/maximum value in df: (7.795, 8.329)
2025-03-04 21:48:44 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:44 Determining energy ratios for test turbine = 006. WD bias: 65.000 deg.
2025-03-04 21:48:44 Determining energy ratios for test turbine = 001. WD bias: 65.000 deg.
2025-03-04 21:48:44 Determining energy ratios for test turbine = 002. WD bias: 65.000 deg.
2025-03-04 21:48:44 Initializing energy ratio inputs.
2025-03-04 21:48:44 Constructing energy table for wd_bias of 70.00 deg.
2025-03-04 21:48:44 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:44 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:44 minimum/maximum value in df: (7.795, 8.329)
2025-03-04 21:48:44 minimum/maximum value in df: (7.795, 8.329)
2025-03-04 21:48:44 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:44 Determining energy ratios for test turbine = 006. WD bias: 70.000 deg.
2025-03-04 21:48:44 Determining energy ratios for test turbine = 001. WD bias: 70.000 deg.
2025-03-04 21:48:45 Determining energy ratios for test turbine = 002. WD bias: 70.000 deg.
2025-03-04 21:48:45 Initializing energy ratio inputs.
2025-03-04 21:48:45 Constructing energy table for wd_bias of 75.00 deg.
2025-03-04 21:48:45 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:45 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:45 minimum/maximum value in df: (7.798, 8.329)
2025-03-04 21:48:45 minimum/maximum value in df: (7.798, 8.329)
2025-03-04 21:48:45 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:45 Determining energy ratios for test turbine = 006. WD bias: 75.000 deg.
2025-03-04 21:48:45 Determining energy ratios for test turbine = 001. WD bias: 75.000 deg.
2025-03-04 21:48:45 Determining energy ratios for test turbine = 002. WD bias: 75.000 deg.
2025-03-04 21:48:45 Initializing energy ratio inputs.
2025-03-04 21:48:45 Constructing energy table for wd_bias of 80.00 deg.
2025-03-04 21:48:45 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:45 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:45 minimum/maximum value in df: (7.798, 8.329)
2025-03-04 21:48:45 minimum/maximum value in df: (7.798, 8.329)
2025-03-04 21:48:45 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:45 Determining energy ratios for test turbine = 006. WD bias: 80.000 deg.
2025-03-04 21:48:45 Determining energy ratios for test turbine = 001. WD bias: 80.000 deg.
2025-03-04 21:48:45 Determining energy ratios for test turbine = 002. WD bias: 80.000 deg.
2025-03-04 21:48:45 Initializing energy ratio inputs.
2025-03-04 21:48:45 Constructing energy table for wd_bias of 85.00 deg.
2025-03-04 21:48:45 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:45 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:45 minimum/maximum value in df: (7.798, 8.235)
2025-03-04 21:48:45 minimum/maximum value in df: (7.798, 8.235)
2025-03-04 21:48:45 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:46 Determining energy ratios for test turbine = 006. WD bias: 85.000 deg.
2025-03-04 21:48:46 Determining energy ratios for test turbine = 001. WD bias: 85.000 deg.
2025-03-04 21:48:46 Determining energy ratios for test turbine = 002. WD bias: 85.000 deg.
2025-03-04 21:48:46 Initializing energy ratio inputs.
2025-03-04 21:48:46 Constructing energy table for wd_bias of 90.00 deg.
2025-03-04 21:48:46 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:46 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:46 minimum/maximum value in df: (7.798, 8.235)
2025-03-04 21:48:46 minimum/maximum value in df: (7.798, 8.235)
2025-03-04 21:48:46 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:46 Determining energy ratios for test turbine = 006. WD bias: 90.000 deg.
2025-03-04 21:48:46 Determining energy ratios for test turbine = 001. WD bias: 90.000 deg.
2025-03-04 21:48:46 Determining energy ratios for test turbine = 002. WD bias: 90.000 deg.
2025-03-04 21:48:46 Initializing energy ratio inputs.
2025-03-04 21:48:46 Constructing energy table for wd_bias of 95.00 deg.
2025-03-04 21:48:46 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:46 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:46 minimum/maximum value in df: (7.789, 8.235)
2025-03-04 21:48:46 minimum/maximum value in df: (7.789, 8.235)
2025-03-04 21:48:46 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:46 Determining energy ratios for test turbine = 006. WD bias: 95.000 deg.
2025-03-04 21:48:46 Determining energy ratios for test turbine = 001. WD bias: 95.000 deg.
2025-03-04 21:48:46 Determining energy ratios for test turbine = 002. WD bias: 95.000 deg.
2025-03-04 21:48:46 Initializing energy ratio inputs.
2025-03-04 21:48:46 Constructing energy table for wd_bias of 100.00 deg.
2025-03-04 21:48:46 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:46 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:46 minimum/maximum value in df: (7.789, 8.204)
2025-03-04 21:48:46 minimum/maximum value in df: (7.789, 8.204)
2025-03-04 21:48:46 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:47 Determining energy ratios for test turbine = 006. WD bias: 100.000 deg.
2025-03-04 21:48:47 Determining energy ratios for test turbine = 001. WD bias: 100.000 deg.
2025-03-04 21:48:47 Determining energy ratios for test turbine = 002. WD bias: 100.000 deg.
2025-03-04 21:48:47 Initializing energy ratio inputs.
2025-03-04 21:48:47 Constructing energy table for wd_bias of 105.00 deg.
2025-03-04 21:48:47 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:47 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:47 minimum/maximum value in df: (7.789, 8.204)
2025-03-04 21:48:47 minimum/maximum value in df: (7.789, 8.204)
2025-03-04 21:48:47 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:47 Determining energy ratios for test turbine = 006. WD bias: 105.000 deg.
2025-03-04 21:48:47 Determining energy ratios for test turbine = 001. WD bias: 105.000 deg.
2025-03-04 21:48:47 Determining energy ratios for test turbine = 002. WD bias: 105.000 deg.
2025-03-04 21:48:47 Initializing energy ratio inputs.
2025-03-04 21:48:47 Constructing energy table for wd_bias of 110.00 deg.
2025-03-04 21:48:47 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:47 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:47 minimum/maximum value in df: (7.776, 8.195)
2025-03-04 21:48:47 minimum/maximum value in df: (7.776, 8.195)
2025-03-04 21:48:47 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:47 Determining energy ratios for test turbine = 006. WD bias: 110.000 deg.
2025-03-04 21:48:47 Determining energy ratios for test turbine = 001. WD bias: 110.000 deg.
2025-03-04 21:48:47 Determining energy ratios for test turbine = 002. WD bias: 110.000 deg.
2025-03-04 21:48:47 Initializing energy ratio inputs.
2025-03-04 21:48:47 Constructing energy table for wd_bias of 115.00 deg.
2025-03-04 21:48:47 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:47 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:47 minimum/maximum value in df: (7.776, 8.224)
2025-03-04 21:48:47 minimum/maximum value in df: (7.776, 8.224)
2025-03-04 21:48:47 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:48 Determining energy ratios for test turbine = 006. WD bias: 115.000 deg.
2025-03-04 21:48:48 Determining energy ratios for test turbine = 001. WD bias: 115.000 deg.
2025-03-04 21:48:48 Determining energy ratios for test turbine = 002. WD bias: 115.000 deg.
2025-03-04 21:48:48 Initializing energy ratio inputs.
2025-03-04 21:48:48 Constructing energy table for wd_bias of 120.00 deg.
2025-03-04 21:48:48 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:48 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:48 minimum/maximum value in df: (7.776, 8.224)
2025-03-04 21:48:48 minimum/maximum value in df: (7.776, 8.224)
2025-03-04 21:48:48 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:48 Determining energy ratios for test turbine = 006. WD bias: 120.000 deg.
2025-03-04 21:48:48 Determining energy ratios for test turbine = 001. WD bias: 120.000 deg.
2025-03-04 21:48:48 Determining energy ratios for test turbine = 002. WD bias: 120.000 deg.
2025-03-04 21:48:48 Initializing energy ratio inputs.
2025-03-04 21:48:48 Constructing energy table for wd_bias of 125.00 deg.
2025-03-04 21:48:48 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:48 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:48 minimum/maximum value in df: (7.776, 8.224)
2025-03-04 21:48:48 minimum/maximum value in df: (7.776, 8.224)
2025-03-04 21:48:48 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:48 Determining energy ratios for test turbine = 006. WD bias: 125.000 deg.
2025-03-04 21:48:48 Determining energy ratios for test turbine = 001. WD bias: 125.000 deg.
2025-03-04 21:48:48 Determining energy ratios for test turbine = 002. WD bias: 125.000 deg.
2025-03-04 21:48:48 Initializing energy ratio inputs.
2025-03-04 21:48:48 Constructing energy table for wd_bias of 130.00 deg.
2025-03-04 21:48:49 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:49 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:49 minimum/maximum value in df: (7.785, 8.227)
2025-03-04 21:48:49 minimum/maximum value in df: (7.785, 8.227)
2025-03-04 21:48:49 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:49 Determining energy ratios for test turbine = 006. WD bias: 130.000 deg.
2025-03-04 21:48:49 Determining energy ratios for test turbine = 001. WD bias: 130.000 deg.
2025-03-04 21:48:49 Determining energy ratios for test turbine = 002. WD bias: 130.000 deg.
2025-03-04 21:48:49 Initializing energy ratio inputs.
2025-03-04 21:48:49 Constructing energy table for wd_bias of 135.00 deg.
2025-03-04 21:48:49 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:49 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:49 minimum/maximum value in df: (7.785, 8.227)
2025-03-04 21:48:49 minimum/maximum value in df: (7.785, 8.227)
2025-03-04 21:48:49 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:49 Determining energy ratios for test turbine = 006. WD bias: 135.000 deg.
2025-03-04 21:48:49 Determining energy ratios for test turbine = 001. WD bias: 135.000 deg.
2025-03-04 21:48:49 Determining energy ratios for test turbine = 002. WD bias: 135.000 deg.
2025-03-04 21:48:49 Initializing energy ratio inputs.
2025-03-04 21:48:49 Constructing energy table for wd_bias of 140.00 deg.
2025-03-04 21:48:49 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:49 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:49 minimum/maximum value in df: (7.785, 8.227)
2025-03-04 21:48:49 minimum/maximum value in df: (7.785, 8.227)
2025-03-04 21:48:49 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:49 Determining energy ratios for test turbine = 006. WD bias: 140.000 deg.
2025-03-04 21:48:49 Determining energy ratios for test turbine = 001. WD bias: 140.000 deg.
2025-03-04 21:48:49 Determining energy ratios for test turbine = 002. WD bias: 140.000 deg.
2025-03-04 21:48:49 Initializing energy ratio inputs.
2025-03-04 21:48:49 Constructing energy table for wd_bias of 145.00 deg.
2025-03-04 21:48:50 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:50 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:50 minimum/maximum value in df: (7.798, 8.256)
2025-03-04 21:48:50 minimum/maximum value in df: (7.798, 8.256)
2025-03-04 21:48:50 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:50 Determining energy ratios for test turbine = 006. WD bias: 145.000 deg.
2025-03-04 21:48:50 Determining energy ratios for test turbine = 001. WD bias: 145.000 deg.
2025-03-04 21:48:50 Determining energy ratios for test turbine = 002. WD bias: 145.000 deg.
2025-03-04 21:48:50 Initializing energy ratio inputs.
2025-03-04 21:48:50 Constructing energy table for wd_bias of 150.00 deg.
2025-03-04 21:48:50 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:50 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:50 minimum/maximum value in df: (7.798, 8.256)
2025-03-04 21:48:50 minimum/maximum value in df: (7.798, 8.256)
2025-03-04 21:48:50 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:50 Determining energy ratios for test turbine = 006. WD bias: 150.000 deg.
2025-03-04 21:48:50 Determining energy ratios for test turbine = 001. WD bias: 150.000 deg.
2025-03-04 21:48:50 Determining energy ratios for test turbine = 002. WD bias: 150.000 deg.
2025-03-04 21:48:50 Initializing energy ratio inputs.
2025-03-04 21:48:50 Constructing energy table for wd_bias of 155.00 deg.
2025-03-04 21:48:50 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:50 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:50 minimum/maximum value in df: (7.804, 8.256)
2025-03-04 21:48:50 minimum/maximum value in df: (7.804, 8.256)
2025-03-04 21:48:50 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:50 Determining energy ratios for test turbine = 006. WD bias: 155.000 deg.
2025-03-04 21:48:50 Determining energy ratios for test turbine = 001. WD bias: 155.000 deg.
2025-03-04 21:48:51 Determining energy ratios for test turbine = 002. WD bias: 155.000 deg.
2025-03-04 21:48:51 Initializing energy ratio inputs.
2025-03-04 21:48:51 Constructing energy table for wd_bias of 160.00 deg.
2025-03-04 21:48:51 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:51 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:51 minimum/maximum value in df: (7.798, 8.256)
2025-03-04 21:48:51 minimum/maximum value in df: (7.798, 8.256)
2025-03-04 21:48:51 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:51 Determining energy ratios for test turbine = 006. WD bias: 160.000 deg.
2025-03-04 21:48:51 Determining energy ratios for test turbine = 001. WD bias: 160.000 deg.
2025-03-04 21:48:51 Determining energy ratios for test turbine = 002. WD bias: 160.000 deg.
2025-03-04 21:48:51 Initializing energy ratio inputs.
2025-03-04 21:48:51 Constructing energy table for wd_bias of 165.00 deg.
2025-03-04 21:48:51 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:51 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:51 minimum/maximum value in df: (7.798, 8.247)
2025-03-04 21:48:51 minimum/maximum value in df: (7.798, 8.247)
2025-03-04 21:48:51 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:51 Determining energy ratios for test turbine = 006. WD bias: 165.000 deg.
2025-03-04 21:48:51 Determining energy ratios for test turbine = 001. WD bias: 165.000 deg.
2025-03-04 21:48:51 Determining energy ratios for test turbine = 002. WD bias: 165.000 deg.
2025-03-04 21:48:51 Initializing energy ratio inputs.
2025-03-04 21:48:51 Constructing energy table for wd_bias of 170.00 deg.
2025-03-04 21:48:51 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:51 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:51 minimum/maximum value in df: (7.798, 8.247)
2025-03-04 21:48:51 minimum/maximum value in df: (7.798, 8.247)
2025-03-04 21:48:51 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:51 Determining energy ratios for test turbine = 006. WD bias: 170.000 deg.
2025-03-04 21:48:52 Determining energy ratios for test turbine = 001. WD bias: 170.000 deg.
2025-03-04 21:48:52 Determining energy ratios for test turbine = 002. WD bias: 170.000 deg.
2025-03-04 21:48:52 Initializing energy ratio inputs.
2025-03-04 21:48:52 Constructing energy table for wd_bias of 175.00 deg.
2025-03-04 21:48:52 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:52 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:52 minimum/maximum value in df: (7.798, 8.247)
2025-03-04 21:48:52 minimum/maximum value in df: (7.798, 8.247)
2025-03-04 21:48:52 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:52 Determining energy ratios for test turbine = 006. WD bias: 175.000 deg.
2025-03-04 21:48:52 Determining energy ratios for test turbine = 001. WD bias: 175.000 deg.
2025-03-04 21:48:52 Determining energy ratios for test turbine = 002. WD bias: 175.000 deg.
2025-03-04 21:48:52 Initializing energy ratio inputs.
2025-03-04 21:48:52 Constructing energy table for wd_bias of 180.00 deg.
2025-03-04 21:48:52 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:52 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:52 minimum/maximum value in df: (7.798, 8.248)
2025-03-04 21:48:52 minimum/maximum value in df: (7.798, 8.248)
2025-03-04 21:48:52 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:52 Determining energy ratios for test turbine = 006. WD bias: 180.000 deg.
2025-03-04 21:48:52 Determining energy ratios for test turbine = 001. WD bias: 180.000 deg.
2025-03-04 21:48:52 Determining energy ratios for test turbine = 002. WD bias: 180.000 deg.
2025-03-04 21:48:52 Initializing energy ratio inputs.
2025-03-04 21:48:52 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:52 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:52 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:52 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:52 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:52 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:53 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:53 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:53 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:48:53 Initializing energy ratio inputs.
2025-03-04 21:48:53 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:53 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:53 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:53 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:53 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:53 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:53 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:53 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:53 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:48:53 Evaluating optimal solution with bootstrapping
2025-03-04 21:48:53 Initializing energy ratio inputs.
2025-03-04 21:48:53 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:53 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:53 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:53 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:53 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:53 minimum/maximum value in df_approx: (8.000, 8.000)
Optimization terminated successfully.
Current function value: -0.893931
Iterations: 1
Function evaluations: 2
2025-03-04 21:48:53 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:53 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:53 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:48:53 Initializing energy ratio inputs.
2025-03-04 21:48:53 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:53 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:53 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:53 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:53 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:53 minimum/maximum value in df_approx: (8.000, 8.000)
Turbine 0. estimated bias = 0.00025 deg.
2025-03-04 21:48:54 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:54 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:54 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
WD bias for first clean turbine: 0.000 deg
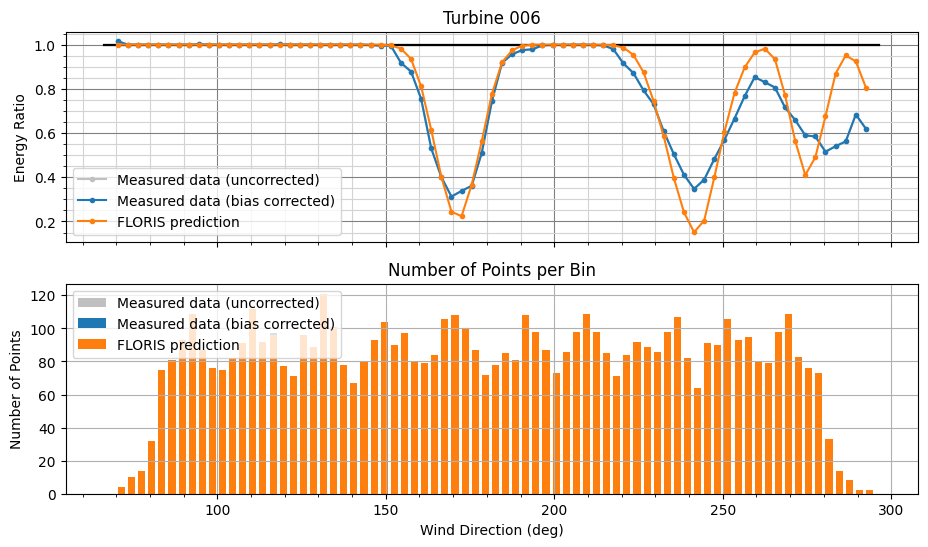
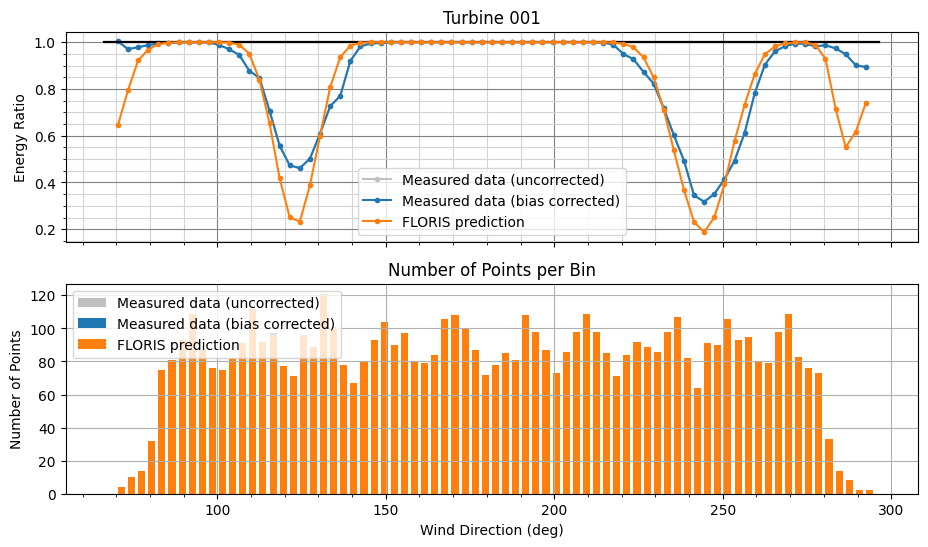
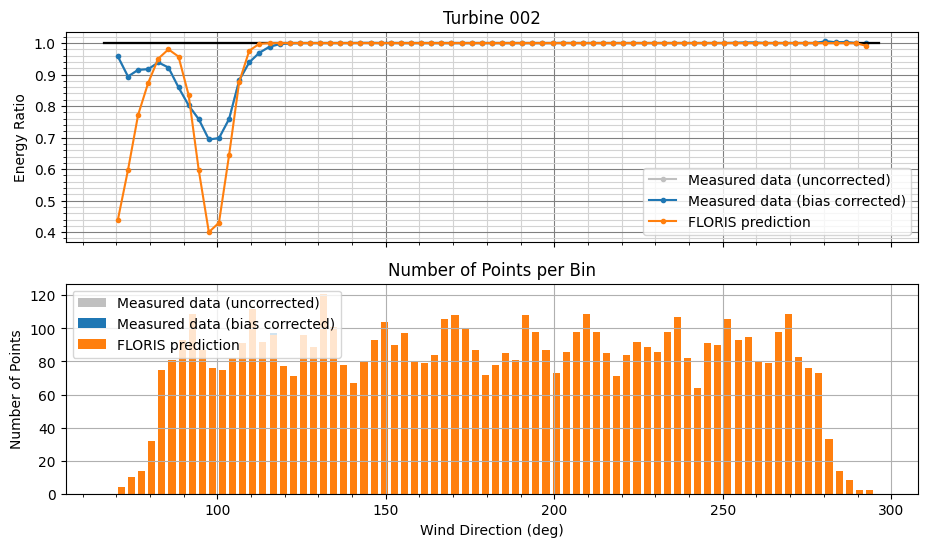
Step 4: Estimate the biases of the remaining turbines#
Now that we know the wind direction bias of a single turbine, we roughly know where true north lies. What we can now do is simply calculate the average offset between the wind direction of any turbine and this northing-calibrated wind direction. The wind direction bias for that turbine is likely very close to this number. We use this as a first guess for the energy-ratio-based bias estimation, and optimize within +- 5 degrees within this initial value.
def estimate_biases_with_reference_wd(df_scada, fm, wd_ref):
# Now use this knowledge to estimate bias for every turbine
num_turbines = len(fm.layout_x)
wd_bias_list = np.zeros(num_turbines)
for ti in range(num_turbines):
# Calculate the offset between this turbine's wind direction and that
# of the calibrated (reference) wind direction. Note that 'wd_ref' may
# also be a met mast' wind direction signal, if available. The offset
# between a turbine's wind direction and wd_ref is very likely to be
# the bias or close to the bias in this turbine's northing.
wd_test = df_scada["wd_{:03d}".format(ti)]
x0, _ = flopt.match_y_curves_by_offset(
wd_ref, wd_test, dy_eval=np.arange(-180.0, 180.0, 2.0), angle_wrapping=True
)
# Then, we refine this first guess by evaluating the cost function
# at [-5.0, 0.0, 5.0] deg around x0, and let the optimizer
# converge.
x_search_bounds = np.round(x0) + np.array([-5.0, 5.0])
# Calculate and save the results to a list
wd_bias_list[ti] = get_bias_for_single_turbine(
df=df_scada,
fm=fm,
ti=ti,
opt_search_range=x_search_bounds,
plot=True,
figure_save_path=None,
)
print(" ")
return wd_bias_list
wd_bias_list = estimate_biases_with_reference_wd(df_scada_homogenized, fm, wd_ref)
print("Wind direction biases: {}".format(wd_bias_list))
2025-03-04 21:48:56 Initializing a bias_estimation() object...
2025-03-04 21:48:56 Estimating the wind direction bias
2025-03-04 21:48:56 Initializing energy ratio inputs.
2025-03-04 21:48:56 Constructing energy table for wd_bias of -5.00 deg.
2025-03-04 21:48:56 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:56 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:56 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:48:56 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:48:56 minimum/maximum value in df_approx: (8.000, 8.000)
Initializing wd bias estimator object for turbine 000...
2025-03-04 21:48:56 Determining energy ratios for test turbine = 006. WD bias: -5.000 deg.
2025-03-04 21:48:56 Determining energy ratios for test turbine = 001. WD bias: -5.000 deg.
2025-03-04 21:48:56 Determining energy ratios for test turbine = 002. WD bias: -5.000 deg.
2025-03-04 21:48:56 Initializing energy ratio inputs.
2025-03-04 21:48:56 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:57 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:57 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:57 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:57 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:57 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:57 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:57 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:57 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:48:57 Initializing energy ratio inputs.
2025-03-04 21:48:57 Constructing energy table for wd_bias of 5.00 deg.
2025-03-04 21:48:57 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:57 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:57 minimum/maximum value in df: (7.789, 8.231)
2025-03-04 21:48:57 minimum/maximum value in df: (7.789, 8.231)
2025-03-04 21:48:57 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:57 Determining energy ratios for test turbine = 006. WD bias: 5.000 deg.
2025-03-04 21:48:57 Determining energy ratios for test turbine = 001. WD bias: 5.000 deg.
2025-03-04 21:48:57 Determining energy ratios for test turbine = 002. WD bias: 5.000 deg.
2025-03-04 21:48:57 Initializing energy ratio inputs.
2025-03-04 21:48:57 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:57 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:57 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:57 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:57 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:57 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:57 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:57 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:57 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:48:57 Initializing energy ratio inputs.
2025-03-04 21:48:58 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:58 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:58 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:58 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:58 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:58 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:48:58 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:58 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:58 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:48:58 Evaluating optimal solution with bootstrapping
2025-03-04 21:48:58 Initializing energy ratio inputs.
2025-03-04 21:48:58 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:58 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:58 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:58 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:58 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:58 minimum/maximum value in df_approx: (8.000, 8.000)
Optimization terminated successfully.
Current function value: -0.893931
Iterations: 1
Function evaluations: 2
2025-03-04 21:48:58 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:58 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:58 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:48:58 Initializing energy ratio inputs.
2025-03-04 21:48:58 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:48:58 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:58 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:58 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:58 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:48:58 minimum/maximum value in df_approx: (8.000, 8.000)
Turbine 0. estimated bias = 0.00025 deg.
2025-03-04 21:48:58 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:48:58 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:48:59 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:48:59 Initializing a bias_estimation() object...
2025-03-04 21:48:59 Estimating the wind direction bias
2025-03-04 21:48:59 Initializing energy ratio inputs.
2025-03-04 21:48:59 Constructing energy table for wd_bias of 9.00 deg.
Initializing wd bias estimator object for turbine 001...
2025-03-04 21:48:59 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:48:59 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:48:59 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:48:59 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:48:59 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:00 Determining energy ratios for test turbine = 002. WD bias: 9.000 deg.
2025-03-04 21:49:00 Determining energy ratios for test turbine = 006. WD bias: 9.000 deg.
2025-03-04 21:49:00 Determining energy ratios for test turbine = 005. WD bias: 9.000 deg.
2025-03-04 21:49:00 Initializing energy ratio inputs.
2025-03-04 21:49:00 Constructing energy table for wd_bias of 14.00 deg.
2025-03-04 21:49:00 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:00 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:00 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:00 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:00 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:00 Determining energy ratios for test turbine = 002. WD bias: 14.000 deg.
2025-03-04 21:49:00 Determining energy ratios for test turbine = 006. WD bias: 14.000 deg.
2025-03-04 21:49:00 Determining energy ratios for test turbine = 005. WD bias: 14.000 deg.
2025-03-04 21:49:00 Initializing energy ratio inputs.
2025-03-04 21:49:00 Constructing energy table for wd_bias of 19.00 deg.
2025-03-04 21:49:00 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:00 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:00 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:00 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:00 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:00 Determining energy ratios for test turbine = 002. WD bias: 19.000 deg.
2025-03-04 21:49:00 Determining energy ratios for test turbine = 006. WD bias: 19.000 deg.
2025-03-04 21:49:00 Determining energy ratios for test turbine = 005. WD bias: 19.000 deg.
2025-03-04 21:49:00 Initializing energy ratio inputs.
2025-03-04 21:49:00 Constructing energy table for wd_bias of 14.00 deg.
2025-03-04 21:49:00 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:00 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:00 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:00 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:00 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:01 Determining energy ratios for test turbine = 002. WD bias: 14.000 deg.
2025-03-04 21:49:01 Determining energy ratios for test turbine = 006. WD bias: 14.000 deg.
2025-03-04 21:49:01 Determining energy ratios for test turbine = 005. WD bias: 14.000 deg.
2025-03-04 21:49:01 Initializing energy ratio inputs.
2025-03-04 21:49:01 Constructing energy table for wd_bias of 14.70 deg.
2025-03-04 21:49:01 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:01 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:01 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:01 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:01 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:01 Determining energy ratios for test turbine = 002. WD bias: 14.700 deg.
2025-03-04 21:49:01 Determining energy ratios for test turbine = 006. WD bias: 14.700 deg.
2025-03-04 21:49:01 Determining energy ratios for test turbine = 005. WD bias: 14.700 deg.
2025-03-04 21:49:01 Initializing energy ratio inputs.
2025-03-04 21:49:01 Constructing energy table for wd_bias of 15.40 deg.
2025-03-04 21:49:01 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:01 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:01 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:01 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:01 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:01 Determining energy ratios for test turbine = 002. WD bias: 15.400 deg.
2025-03-04 21:49:01 Determining energy ratios for test turbine = 006. WD bias: 15.400 deg.
2025-03-04 21:49:01 Determining energy ratios for test turbine = 005. WD bias: 15.400 deg.
2025-03-04 21:49:01 Initializing energy ratio inputs.
2025-03-04 21:49:01 Constructing energy table for wd_bias of 15.05 deg.
2025-03-04 21:49:01 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:01 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:01 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:01 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:01 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:02 Determining energy ratios for test turbine = 002. WD bias: 15.050 deg.
2025-03-04 21:49:02 Determining energy ratios for test turbine = 006. WD bias: 15.050 deg.
2025-03-04 21:49:02 Determining energy ratios for test turbine = 005. WD bias: 15.050 deg.
2025-03-04 21:49:02 Initializing energy ratio inputs.
2025-03-04 21:49:02 Constructing energy table for wd_bias of 15.40 deg.
2025-03-04 21:49:02 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:02 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:02 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:02 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:02 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:02 Determining energy ratios for test turbine = 002. WD bias: 15.400 deg.
2025-03-04 21:49:02 Determining energy ratios for test turbine = 006. WD bias: 15.400 deg.
2025-03-04 21:49:02 Determining energy ratios for test turbine = 005. WD bias: 15.400 deg.
2025-03-04 21:49:02 Initializing energy ratio inputs.
2025-03-04 21:49:02 Constructing energy table for wd_bias of 14.88 deg.
2025-03-04 21:49:02 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:02 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:02 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:02 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:02 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:02 Determining energy ratios for test turbine = 002. WD bias: 14.875 deg.
2025-03-04 21:49:02 Determining energy ratios for test turbine = 006. WD bias: 14.875 deg.
2025-03-04 21:49:02 Determining energy ratios for test turbine = 005. WD bias: 14.875 deg.
2025-03-04 21:49:02 Initializing energy ratio inputs.
2025-03-04 21:49:02 Constructing energy table for wd_bias of 15.23 deg.
2025-03-04 21:49:03 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:03 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:03 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:03 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:03 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:03 Determining energy ratios for test turbine = 002. WD bias: 15.225 deg.
2025-03-04 21:49:03 Determining energy ratios for test turbine = 006. WD bias: 15.225 deg.
2025-03-04 21:49:03 Determining energy ratios for test turbine = 005. WD bias: 15.225 deg.
2025-03-04 21:49:03 Initializing energy ratio inputs.
2025-03-04 21:49:03 Constructing energy table for wd_bias of 14.96 deg.
2025-03-04 21:49:03 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:03 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:03 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:03 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:03 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:03 Determining energy ratios for test turbine = 002. WD bias: 14.963 deg.
2025-03-04 21:49:03 Determining energy ratios for test turbine = 006. WD bias: 14.963 deg.
2025-03-04 21:49:03 Determining energy ratios for test turbine = 005. WD bias: 14.963 deg.
2025-03-04 21:49:03 Evaluating optimal solution with bootstrapping
2025-03-04 21:49:03 Initializing energy ratio inputs.
2025-03-04 21:49:03 Constructing energy table for wd_bias of 14.96 deg.
2025-03-04 21:49:03 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:03 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:03 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:03 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:03 minimum/maximum value in df_approx: (8.000, 8.000)
Optimization terminated successfully.
Current function value: -0.999869
Iterations: 4
Function evaluations: 8
2025-03-04 21:49:03 Determining energy ratios for test turbine = 002. WD bias: 14.963 deg.
2025-03-04 21:49:03 Determining energy ratios for test turbine = 006. WD bias: 14.963 deg.
2025-03-04 21:49:03 Determining energy ratios for test turbine = 005. WD bias: 14.963 deg.
2025-03-04 21:49:04 Initializing energy ratio inputs.
2025-03-04 21:49:04 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:04 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:04 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:04 minimum/maximum value in df: (7.776, 8.253)
2025-03-04 21:49:04 minimum/maximum value in df: (7.776, 8.253)
2025-03-04 21:49:04 minimum/maximum value in df_approx: (8.000, 8.000)
Turbine 1. estimated bias = 14.9625 deg.
2025-03-04 21:49:04 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:04 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:49:04 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:05 Initializing a bias_estimation() object...
2025-03-04 21:49:05 Estimating the wind direction bias
2025-03-04 21:49:05 Initializing energy ratio inputs.
2025-03-04 21:49:05 Constructing energy table for wd_bias of -49.00 deg.
Initializing wd bias estimator object for turbine 002...
2025-03-04 21:49:05 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:05 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:05 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:05 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:05 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:05 Determining energy ratios for test turbine = 001. WD bias: -49.000 deg.
2025-03-04 21:49:05 Determining energy ratios for test turbine = 003. WD bias: -49.000 deg.
2025-03-04 21:49:05 Determining energy ratios for test turbine = 005. WD bias: -49.000 deg.
2025-03-04 21:49:05 Initializing energy ratio inputs.
2025-03-04 21:49:05 Constructing energy table for wd_bias of -44.00 deg.
2025-03-04 21:49:05 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:05 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:05 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:05 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:05 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:05 Determining energy ratios for test turbine = 001. WD bias: -44.000 deg.
2025-03-04 21:49:05 Determining energy ratios for test turbine = 003. WD bias: -44.000 deg.
2025-03-04 21:49:05 Determining energy ratios for test turbine = 005. WD bias: -44.000 deg.
2025-03-04 21:49:05 Initializing energy ratio inputs.
2025-03-04 21:49:05 Constructing energy table for wd_bias of -39.00 deg.
2025-03-04 21:49:05 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:05 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:05 minimum/maximum value in df: (7.787, 8.231)
2025-03-04 21:49:05 minimum/maximum value in df: (7.787, 8.231)
2025-03-04 21:49:05 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:06 Determining energy ratios for test turbine = 001. WD bias: -39.000 deg.
2025-03-04 21:49:06 Determining energy ratios for test turbine = 003. WD bias: -39.000 deg.
2025-03-04 21:49:06 Determining energy ratios for test turbine = 005. WD bias: -39.000 deg.
2025-03-04 21:49:06 Initializing energy ratio inputs.
2025-03-04 21:49:06 Constructing energy table for wd_bias of -44.00 deg.
2025-03-04 21:49:06 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:06 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:06 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:06 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:06 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:06 Determining energy ratios for test turbine = 001. WD bias: -44.000 deg.
2025-03-04 21:49:06 Determining energy ratios for test turbine = 003. WD bias: -44.000 deg.
2025-03-04 21:49:06 Determining energy ratios for test turbine = 005. WD bias: -44.000 deg.
2025-03-04 21:49:06 Initializing energy ratio inputs.
2025-03-04 21:49:06 Constructing energy table for wd_bias of -46.20 deg.
2025-03-04 21:49:06 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:06 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:06 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:06 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:06 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:06 Determining energy ratios for test turbine = 001. WD bias: -46.200 deg.
2025-03-04 21:49:06 Determining energy ratios for test turbine = 003. WD bias: -46.200 deg.
2025-03-04 21:49:06 Determining energy ratios for test turbine = 005. WD bias: -46.200 deg.
2025-03-04 21:49:06 Initializing energy ratio inputs.
2025-03-04 21:49:06 Constructing energy table for wd_bias of -41.80 deg.
2025-03-04 21:49:06 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:06 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:06 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:06 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:06 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:07 Determining energy ratios for test turbine = 001. WD bias: -41.800 deg.
2025-03-04 21:49:07 Determining energy ratios for test turbine = 003. WD bias: -41.800 deg.
2025-03-04 21:49:07 Determining energy ratios for test turbine = 005. WD bias: -41.800 deg.
2025-03-04 21:49:07 Initializing energy ratio inputs.
2025-03-04 21:49:07 Constructing energy table for wd_bias of -45.10 deg.
2025-03-04 21:49:07 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:07 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:07 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:07 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:07 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:07 Determining energy ratios for test turbine = 001. WD bias: -45.100 deg.
2025-03-04 21:49:07 Determining energy ratios for test turbine = 003. WD bias: -45.100 deg.
2025-03-04 21:49:07 Determining energy ratios for test turbine = 005. WD bias: -45.100 deg.
2025-03-04 21:49:07 Initializing energy ratio inputs.
2025-03-04 21:49:07 Constructing energy table for wd_bias of -46.20 deg.
2025-03-04 21:49:07 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:07 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:07 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:07 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:07 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:07 Determining energy ratios for test turbine = 001. WD bias: -46.200 deg.
2025-03-04 21:49:07 Determining energy ratios for test turbine = 003. WD bias: -46.200 deg.
2025-03-04 21:49:07 Determining energy ratios for test turbine = 005. WD bias: -46.200 deg.
2025-03-04 21:49:07 Initializing energy ratio inputs.
2025-03-04 21:49:07 Constructing energy table for wd_bias of -44.55 deg.
2025-03-04 21:49:07 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:07 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:07 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:07 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:07 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:08 Determining energy ratios for test turbine = 001. WD bias: -44.550 deg.
2025-03-04 21:49:08 Determining energy ratios for test turbine = 003. WD bias: -44.550 deg.
2025-03-04 21:49:08 Determining energy ratios for test turbine = 005. WD bias: -44.550 deg.
2025-03-04 21:49:08 Initializing energy ratio inputs.
2025-03-04 21:49:08 Constructing energy table for wd_bias of -45.65 deg.
2025-03-04 21:49:08 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:08 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:08 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:08 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:08 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:08 Determining energy ratios for test turbine = 001. WD bias: -45.650 deg.
2025-03-04 21:49:08 Determining energy ratios for test turbine = 003. WD bias: -45.650 deg.
2025-03-04 21:49:08 Determining energy ratios for test turbine = 005. WD bias: -45.650 deg.
2025-03-04 21:49:08 Initializing energy ratio inputs.
2025-03-04 21:49:08 Constructing energy table for wd_bias of -44.83 deg.
2025-03-04 21:49:08 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:08 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:08 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:08 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:08 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:08 Determining energy ratios for test turbine = 001. WD bias: -44.825 deg.
2025-03-04 21:49:08 Determining energy ratios for test turbine = 003. WD bias: -44.825 deg.
2025-03-04 21:49:08 Determining energy ratios for test turbine = 005. WD bias: -44.825 deg.
2025-03-04 21:49:08 Initializing energy ratio inputs.
2025-03-04 21:49:08 Constructing energy table for wd_bias of -45.38 deg.
2025-03-04 21:49:09 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:09 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:09 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:09 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:09 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:09 Determining energy ratios for test turbine = 001. WD bias: -45.375 deg.
2025-03-04 21:49:09 Determining energy ratios for test turbine = 003. WD bias: -45.375 deg.
2025-03-04 21:49:09 Determining energy ratios for test turbine = 005. WD bias: -45.375 deg.
2025-03-04 21:49:09 Initializing energy ratio inputs.
2025-03-04 21:49:09 Constructing energy table for wd_bias of -44.96 deg.
2025-03-04 21:49:09 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:09 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:09 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:09 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:09 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:09 Determining energy ratios for test turbine = 001. WD bias: -44.963 deg.
2025-03-04 21:49:09 Determining energy ratios for test turbine = 003. WD bias: -44.963 deg.
2025-03-04 21:49:09 Determining energy ratios for test turbine = 005. WD bias: -44.963 deg.
2025-03-04 21:49:09 Evaluating optimal solution with bootstrapping
2025-03-04 21:49:09 Initializing energy ratio inputs.
2025-03-04 21:49:09 Constructing energy table for wd_bias of -44.96 deg.
2025-03-04 21:49:09 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:09 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:09 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:09 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:09 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:09 Determining energy ratios for test turbine = 001. WD bias: -44.963 deg.
2025-03-04 21:49:09 Determining energy ratios for test turbine = 003. WD bias: -44.963 deg.
2025-03-04 21:49:09 Determining energy ratios for test turbine = 005. WD bias: -44.963 deg.
2025-03-04 21:49:10 Initializing energy ratio inputs.
2025-03-04 21:49:10 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:10 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:10 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:10 minimum/maximum value in df: (7.802, 8.239)
2025-03-04 21:49:10 minimum/maximum value in df: (7.802, 8.239)
2025-03-04 21:49:10 minimum/maximum value in df_approx: (8.000, 8.000)
Turbine 2. estimated bias = -44.962500000000006 deg.
2025-03-04 21:49:10 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:10 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:10 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:11 Initializing a bias_estimation() object...
2025-03-04 21:49:11 Estimating the wind direction bias
2025-03-04 21:49:11 Initializing energy ratio inputs.
2025-03-04 21:49:11 Constructing energy table for wd_bias of -5.00 deg.
Initializing wd bias estimator object for turbine 003...
2025-03-04 21:49:11 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:11 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:11 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:11 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:11 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:11 Determining energy ratios for test turbine = 005. WD bias: -5.000 deg.
2025-03-04 21:49:11 Determining energy ratios for test turbine = 002. WD bias: -5.000 deg.
2025-03-04 21:49:11 Determining energy ratios for test turbine = 001. WD bias: -5.000 deg.
2025-03-04 21:49:11 Initializing energy ratio inputs.
2025-03-04 21:49:11 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:11 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:11 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:11 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:11 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:11 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:11 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:11 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:11 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:11 Initializing energy ratio inputs.
2025-03-04 21:49:11 Constructing energy table for wd_bias of 5.00 deg.
2025-03-04 21:49:11 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:11 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:11 minimum/maximum value in df: (7.787, 8.231)
2025-03-04 21:49:11 minimum/maximum value in df: (7.787, 8.231)
2025-03-04 21:49:11 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:12 Determining energy ratios for test turbine = 005. WD bias: 5.000 deg.
2025-03-04 21:49:12 Determining energy ratios for test turbine = 002. WD bias: 5.000 deg.
2025-03-04 21:49:12 Determining energy ratios for test turbine = 001. WD bias: 5.000 deg.
2025-03-04 21:49:12 Initializing energy ratio inputs.
2025-03-04 21:49:12 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:12 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:12 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:12 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:12 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:12 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:12 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:12 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:12 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:12 Initializing energy ratio inputs.
2025-03-04 21:49:12 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:12 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:12 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:12 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:12 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:12 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:12 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:12 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:12 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:12 Evaluating optimal solution with bootstrapping
2025-03-04 21:49:12 Initializing energy ratio inputs.
2025-03-04 21:49:12 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:12 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:12 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:12 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:12 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:12 minimum/maximum value in df_approx: (8.000, 8.000)
Optimization terminated successfully.
Current function value: -0.861142
Iterations: 1
Function evaluations: 2
2025-03-04 21:49:13 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:13 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:13 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:13 Initializing energy ratio inputs.
2025-03-04 21:49:13 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:13 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:13 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:13 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:13 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:13 minimum/maximum value in df_approx: (8.000, 8.000)
Turbine 3. estimated bias = 0.0 deg.
2025-03-04 21:49:13 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:13 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:13 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:14 Initializing a bias_estimation() object...
2025-03-04 21:49:14 Estimating the wind direction bias
2025-03-04 21:49:14 Initializing energy ratio inputs.
2025-03-04 21:49:14 Constructing energy table for wd_bias of -5.00 deg.
Initializing wd bias estimator object for turbine 004...
2025-03-04 21:49:14 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:14 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:14 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:14 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:14 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:14 Determining energy ratios for test turbine = 003. WD bias: -5.000 deg.
2025-03-04 21:49:14 Determining energy ratios for test turbine = 002. WD bias: -5.000 deg.
2025-03-04 21:49:14 Determining energy ratios for test turbine = 005. WD bias: -5.000 deg.
2025-03-04 21:49:14 Initializing energy ratio inputs.
2025-03-04 21:49:14 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:14 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:14 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:14 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:14 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:14 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:14 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:14 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:14 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:14 Initializing energy ratio inputs.
2025-03-04 21:49:14 Constructing energy table for wd_bias of 5.00 deg.
2025-03-04 21:49:14 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:14 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:14 minimum/maximum value in df: (7.776, 8.221)
2025-03-04 21:49:14 minimum/maximum value in df: (7.776, 8.221)
2025-03-04 21:49:14 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:15 Determining energy ratios for test turbine = 003. WD bias: 5.000 deg.
2025-03-04 21:49:15 Determining energy ratios for test turbine = 002. WD bias: 5.000 deg.
2025-03-04 21:49:15 Determining energy ratios for test turbine = 005. WD bias: 5.000 deg.
2025-03-04 21:49:15 Initializing energy ratio inputs.
2025-03-04 21:49:15 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:15 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:15 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:15 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:15 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:15 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:15 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:15 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:15 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:15 Initializing energy ratio inputs.
2025-03-04 21:49:15 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:15 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:15 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:15 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:15 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:15 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:15 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:15 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:15 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:15 Evaluating optimal solution with bootstrapping
2025-03-04 21:49:15 Initializing energy ratio inputs.
2025-03-04 21:49:15 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:16 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:16 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:16 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:16 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:16 minimum/maximum value in df_approx: (8.000, 8.000)
Optimization terminated successfully.
Current function value: -0.890642
Iterations: 1
Function evaluations: 2
2025-03-04 21:49:16 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:16 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:16 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:16 Initializing energy ratio inputs.
2025-03-04 21:49:16 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:16 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:16 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:16 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:16 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:16 minimum/maximum value in df_approx: (8.000, 8.000)
Turbine 4. estimated bias = 0.0 deg.
2025-03-04 21:49:16 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:16 Determining energy ratios for test turbine = 002. WD bias: 0.000 deg.
2025-03-04 21:49:16 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:17 Initializing a bias_estimation() object...
2025-03-04 21:49:17 Estimating the wind direction bias
2025-03-04 21:49:17 Initializing energy ratio inputs.
2025-03-04 21:49:17 Constructing energy table for wd_bias of -5.00 deg.
Initializing wd bias estimator object for turbine 005...
2025-03-04 21:49:17 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:17 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:17 minimum/maximum value in df: (7.776, 8.253)
2025-03-04 21:49:17 minimum/maximum value in df: (7.776, 8.253)
2025-03-04 21:49:17 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:17 Determining energy ratios for test turbine = 003. WD bias: -5.000 deg.
2025-03-04 21:49:17 Determining energy ratios for test turbine = 001. WD bias: -5.000 deg.
2025-03-04 21:49:17 Determining energy ratios for test turbine = 006. WD bias: -5.000 deg.
2025-03-04 21:49:17 Initializing energy ratio inputs.
2025-03-04 21:49:17 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:17 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:17 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:17 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:17 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:17 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:18 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:18 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:18 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:49:18 Initializing energy ratio inputs.
2025-03-04 21:49:18 Constructing energy table for wd_bias of 5.00 deg.
2025-03-04 21:49:18 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:18 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:18 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:18 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:18 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:18 Determining energy ratios for test turbine = 003. WD bias: 5.000 deg.
2025-03-04 21:49:18 Determining energy ratios for test turbine = 001. WD bias: 5.000 deg.
2025-03-04 21:49:18 Determining energy ratios for test turbine = 006. WD bias: 5.000 deg.
2025-03-04 21:49:18 Initializing energy ratio inputs.
2025-03-04 21:49:18 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:18 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:18 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:18 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:18 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:18 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:18 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:18 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:18 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:49:18 Initializing energy ratio inputs.
2025-03-04 21:49:18 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:18 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:18 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:18 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:18 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:18 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:19 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:19 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:19 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:49:19 Evaluating optimal solution with bootstrapping
2025-03-04 21:49:19 Initializing energy ratio inputs.
2025-03-04 21:49:19 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:19 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:19 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:19 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:19 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:19 minimum/maximum value in df_approx: (8.000, 8.000)
Optimization terminated successfully.
Current function value: -0.900443
Iterations: 1
Function evaluations: 2
2025-03-04 21:49:19 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:19 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:19 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:49:19 Initializing energy ratio inputs.
2025-03-04 21:49:19 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:19 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:19 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:19 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:19 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:19 minimum/maximum value in df_approx: (8.000, 8.000)
Turbine 5. estimated bias = 0.0 deg.
2025-03-04 21:49:19 Determining energy ratios for test turbine = 003. WD bias: 0.000 deg.
2025-03-04 21:49:19 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:19 Determining energy ratios for test turbine = 006. WD bias: 0.000 deg.
2025-03-04 21:49:20 Initializing a bias_estimation() object...
2025-03-04 21:49:20 Estimating the wind direction bias
2025-03-04 21:49:20 Initializing energy ratio inputs.
2025-03-04 21:49:20 Constructing energy table for wd_bias of -5.00 deg.
Initializing wd bias estimator object for turbine 006...
2025-03-04 21:49:20 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:20 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:20 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:20 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:20 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:20 Determining energy ratios for test turbine = 001. WD bias: -5.000 deg.
2025-03-04 21:49:20 Determining energy ratios for test turbine = 005. WD bias: -5.000 deg.
2025-03-04 21:49:20 Determining energy ratios for test turbine = 000. WD bias: -5.000 deg.
2025-03-04 21:49:20 Initializing energy ratio inputs.
2025-03-04 21:49:20 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:20 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:20 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:20 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:20 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:20 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:21 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:21 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:21 Determining energy ratios for test turbine = 000. WD bias: 0.000 deg.
2025-03-04 21:49:21 Initializing energy ratio inputs.
2025-03-04 21:49:21 Constructing energy table for wd_bias of 5.00 deg.
2025-03-04 21:49:21 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:21 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:21 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:21 minimum/maximum value in df: (7.776, 8.231)
2025-03-04 21:49:21 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:21 Determining energy ratios for test turbine = 001. WD bias: 5.000 deg.
2025-03-04 21:49:21 Determining energy ratios for test turbine = 005. WD bias: 5.000 deg.
2025-03-04 21:49:21 Determining energy ratios for test turbine = 000. WD bias: 5.000 deg.
2025-03-04 21:49:21 Initializing energy ratio inputs.
2025-03-04 21:49:21 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:21 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:21 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:21 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:21 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:21 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:21 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:21 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:21 Determining energy ratios for test turbine = 000. WD bias: 0.000 deg.
2025-03-04 21:49:21 Initializing energy ratio inputs.
2025-03-04 21:49:21 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:22 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:22 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:22 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:22 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:22 minimum/maximum value in df_approx: (8.000, 8.000)
2025-03-04 21:49:22 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:22 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:22 Determining energy ratios for test turbine = 000. WD bias: 0.000 deg.
2025-03-04 21:49:22 Evaluating optimal solution with bootstrapping
2025-03-04 21:49:22 Initializing energy ratio inputs.
2025-03-04 21:49:22 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:22 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:22 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:22 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:22 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:22 minimum/maximum value in df_approx: (8.000, 8.000)
Optimization terminated successfully.
Current function value: -0.884975
Iterations: 1
Function evaluations: 2
2025-03-04 21:49:22 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:22 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:22 Determining energy ratios for test turbine = 000. WD bias: 0.000 deg.
2025-03-04 21:49:22 Initializing energy ratio inputs.
2025-03-04 21:49:22 Constructing energy table for wd_bias of 0.00 deg.
2025-03-04 21:49:22 Interpolating FLORIS predictions for dataframe.
2025-03-04 21:49:22 Warning: the values in df[ws] exceed the range in the precalculated solutions df_fi_approx[ws].
2025-03-04 21:49:22 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:22 minimum/maximum value in df: (7.776, 8.248)
2025-03-04 21:49:22 minimum/maximum value in df_approx: (8.000, 8.000)
Turbine 6. estimated bias = 0.00025 deg.
2025-03-04 21:49:22 Determining energy ratios for test turbine = 001. WD bias: 0.000 deg.
2025-03-04 21:49:22 Determining energy ratios for test turbine = 005. WD bias: 0.000 deg.
2025-03-04 21:49:22 Determining energy ratios for test turbine = 000. WD bias: 0.000 deg.
Wind direction biases: [ 2.50000e-04 1.49625e+01 -4.49625e+01 0.00000e+00 0.00000e+00
0.00000e+00 2.50000e-04]
Error in callback <function _draw_all_if_interactive at 0x7fc66fbfd510> (for post_execute), with arguments args (),kwargs {}:
---------------------------------------------------------------------------
KeyboardInterrupt Traceback (most recent call last)
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/pyplot.py:279, in _draw_all_if_interactive()
277 def _draw_all_if_interactive() -> None:
278 if matplotlib.is_interactive():
--> 279 draw_all()
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/_pylab_helpers.py:131, in Gcf.draw_all(cls, force)
129 for manager in cls.get_all_fig_managers():
130 if force or manager.canvas.figure.stale:
--> 131 manager.canvas.draw_idle()
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/backend_bases.py:1891, in FigureCanvasBase.draw_idle(self, *args, **kwargs)
1889 if not self._is_idle_drawing:
1890 with self._idle_draw_cntx():
-> 1891 self.draw(*args, **kwargs)
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/backends/backend_agg.py:382, in FigureCanvasAgg.draw(self)
379 # Acquire a lock on the shared font cache.
380 with (self.toolbar._wait_cursor_for_draw_cm() if self.toolbar
381 else nullcontext()):
--> 382 self.figure.draw(self.renderer)
383 # A GUI class may be need to update a window using this draw, so
384 # don't forget to call the superclass.
385 super().draw()
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/artist.py:94, in _finalize_rasterization.<locals>.draw_wrapper(artist, renderer, *args, **kwargs)
92 @wraps(draw)
93 def draw_wrapper(artist, renderer, *args, **kwargs):
---> 94 result = draw(artist, renderer, *args, **kwargs)
95 if renderer._rasterizing:
96 renderer.stop_rasterizing()
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/artist.py:71, in allow_rasterization.<locals>.draw_wrapper(artist, renderer)
68 if artist.get_agg_filter() is not None:
69 renderer.start_filter()
---> 71 return draw(artist, renderer)
72 finally:
73 if artist.get_agg_filter() is not None:
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/figure.py:3257, in Figure.draw(self, renderer)
3254 # ValueError can occur when resizing a window.
3256 self.patch.draw(renderer)
-> 3257 mimage._draw_list_compositing_images(
3258 renderer, self, artists, self.suppressComposite)
3260 renderer.close_group('figure')
3261 finally:
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/image.py:134, in _draw_list_compositing_images(renderer, parent, artists, suppress_composite)
132 if not_composite or not has_images:
133 for a in artists:
--> 134 a.draw(renderer)
135 else:
136 # Composite any adjacent images together
137 image_group = []
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/artist.py:71, in allow_rasterization.<locals>.draw_wrapper(artist, renderer)
68 if artist.get_agg_filter() is not None:
69 renderer.start_filter()
---> 71 return draw(artist, renderer)
72 finally:
73 if artist.get_agg_filter() is not None:
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/axes/_base.py:3210, in _AxesBase.draw(self, renderer)
3207 if artists_rasterized:
3208 _draw_rasterized(self.get_figure(root=True), artists_rasterized, renderer)
-> 3210 mimage._draw_list_compositing_images(
3211 renderer, self, artists, self.get_figure(root=True).suppressComposite)
3213 renderer.close_group('axes')
3214 self.stale = False
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/image.py:134, in _draw_list_compositing_images(renderer, parent, artists, suppress_composite)
132 if not_composite or not has_images:
133 for a in artists:
--> 134 a.draw(renderer)
135 else:
136 # Composite any adjacent images together
137 image_group = []
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/artist.py:71, in allow_rasterization.<locals>.draw_wrapper(artist, renderer)
68 if artist.get_agg_filter() is not None:
69 renderer.start_filter()
---> 71 return draw(artist, renderer)
72 finally:
73 if artist.get_agg_filter() is not None:
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/axis.py:1411, in Axis.draw(self, renderer)
1408 tick.draw(renderer)
1410 # Shift label away from axes to avoid overlapping ticklabels.
-> 1411 self._update_label_position(renderer)
1412 self.label.draw(renderer)
1414 self._update_offset_text_position(tlb1, tlb2)
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/axis.py:2448, in XAxis._update_label_position(self, renderer)
2444 return
2446 # get bounding boxes for this axis and any siblings
2447 # that have been set by `fig.align_xlabels()`
-> 2448 bboxes, bboxes2 = self._get_tick_boxes_siblings(renderer=renderer)
2449 x, y = self.label.get_position()
2451 if self.label_position == 'bottom':
2452 # Union with extents of the bottom spine if present, of the axes otherwise.
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/axis.py:2231, in Axis._get_tick_boxes_siblings(self, renderer)
2224 """
2225 Get the bounding boxes for this `.axis` and its siblings
2226 as set by `.Figure.align_xlabels` or `.Figure.align_ylabels`.
2227
2228 By default, it just gets bboxes for *self*.
2229 """
2230 # Get the Grouper keeping track of x or y label groups for this figure.
-> 2231 name = self._get_axis_name()
2232 if name not in self.get_figure(root=False)._align_label_groups:
2233 return [], []
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/axis.py:713, in Axis._get_axis_name(self)
711 def _get_axis_name(self):
712 """Return the axis name."""
--> 713 return next(name for name, axis in self.axes._axis_map.items()
714 if axis is self)
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/axes/_base.py:602, in _AxesBase._axis_map(self)
599 @property
600 def _axis_map(self):
601 """A mapping of axis names, e.g. 'x', to `Axis` instances."""
--> 602 return {name: getattr(self, f"{name}axis")
603 for name in self._axis_names}
File /opt/hostedtoolcache/Python/3.10.16/x64/lib/python3.10/site-packages/matplotlib/axes/_base.py:602, in <dictcomp>(.0)
599 @property
600 def _axis_map(self):
601 """A mapping of axis names, e.g. 'x', to `Axis` instances."""
--> 602 return {name: getattr(self, f"{name}axis")
603 for name in self._axis_names}
KeyboardInterrupt:
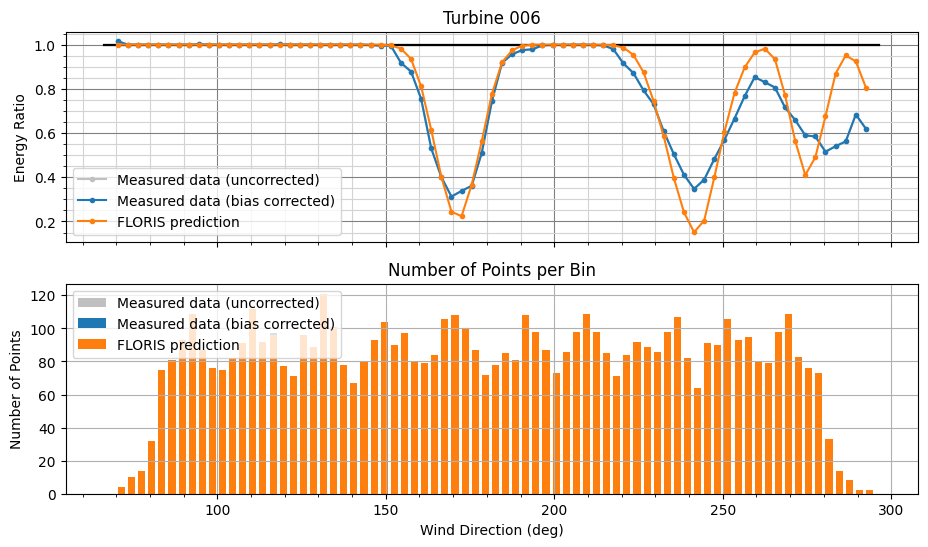
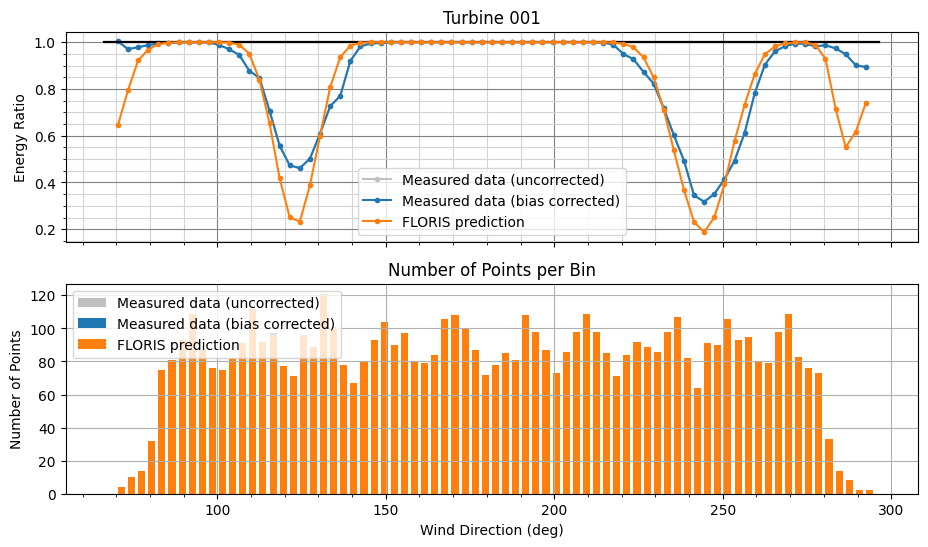
Step 5: Correct turbine wind directions for bias#
The next step is to apply the northing corrections directly on the data.
def apply_bias_corrections(df_scada, wd_bias_list):
# Copy dataframe
df_out = df_scada.copy()
# Load the SCADA data
num_turbines = dfm.get_num_turbines(df_scada)
# Set turbine-individual bias corrections
for ti in range(num_turbines):
bias = wd_bias_list[ti]
print("Removing {:.2f} deg bias for ti = {:03d}.".format(bias, ti))
df_out["wd_{:03d}".format(ti)] = wrap_360(df_out["wd_{:03d}".format(ti)] - bias)
return df_out
# Get bias corrections
print("wd_bias_list: {}".format(wd_bias_list))
df_scada_homogenized = apply_bias_corrections(
df_scada=df_scada_homogenized.copy(), wd_bias_list=wd_bias_list
)
wd_bias_list: [ 2.50000e-04 1.49625e+01 -4.49625e+01 0.00000e+00 0.00000e+00
0.00000e+00 2.50000e-04]
Removing 0.00 deg bias for ti = 000.
Removing 14.96 deg bias for ti = 001.
Removing -44.96 deg bias for ti = 002.
Removing 0.00 deg bias for ti = 003.
Removing 0.00 deg bias for ti = 004.
Removing 0.00 deg bias for ti = 005.
Removing 0.00 deg bias for ti = 006.
Step 6: Deal with inter-turbine faults#
Deal with faults at one turbine causing issues at another turbine. For example, if a turbine is shedding a wake on a second turbine, then for a fair comparison both of these two turbines should be operating normally. If the upstream turbine is curtailed or offline, the power production of the downstream turbine also changes. Hence, if we are unsure about the operating mode of one machine, we cannot make accurate FLORIS predictions on the second turbine either. In this scenario, we would classify the second turbine's measurement as faulty too, because of this.
def filter_for_faults_in_impacting_turbines(df):
# Determine which turbines impact which other turbines through their wakes
print("Calculating the 'df_impacting_turbines' matrix...")
df_impacting_turbines = ftools.get_all_impacting_turbines(
fm_in=fm,
wd_array=np.arange(0.0, 360.0, 3.0),
change_threshold=0.005,
ws_test=9.0,
)
print(df_impacting_turbines)
# Filter the measurements for each turbine: make sure all
# other turbines affecting this turbine's
# power production are marked as good measurements. If they are not,
# then classify this turbine's
# measurement as faulty too.
num_turbines = dfm.get_num_turbines(df)
for ti in range(num_turbines):
# Assign a reference wind direction for this turbine. In this case,
# we have such a small farm so we assume that the farm average wind
# direction of representative of every turbine.
df = dfm.set_wd_by_all_turbines(df)
df_scada = filt.filter_df_by_faulty_impacting_turbines(
df=df,
ti=ti,
df_impacting_turbines=df_impacting_turbines,
verbose=True,
)
return df_scada
df_scada_northing_calibrated_interturbine_filtered = filter_for_faults_in_impacting_turbines(
df=df_scada_homogenized.copy()
)
floris.floris_model.FlorisModel WARNING Deleting stored wind_data information.
Calculating the 'df_impacting_turbines' matrix...
floris.floris_model.FlorisModel WARNING Deleting stored wind_data information.
floris.floris_model.FlorisModel WARNING Deleting stored wind_data information.
floris.floris_model.FlorisModel WARNING Deleting stored wind_data information.
floris.floris_model.FlorisModel WARNING Deleting stored wind_data information.
floris.floris_model.FlorisModel WARNING Deleting stored wind_data information.
floris.floris_model.FlorisModel WARNING Deleting stored wind_data information.
2024-12-02 11:23:51 Faulty measurements for WTG 00 increased from 0.000 % to 0.000 %. Reason: 'Turbine is impacted by faulty upstream turbine'.
2024-12-02 11:23:51 Faulty measurements for WTG 01 increased from 0.000 % to 0.000 %. Reason: 'Turbine is impacted by faulty upstream turbine'.
2024-12-02 11:23:51 Faulty measurements for WTG 02 increased from 0.000 % to 0.000 %. Reason: 'Turbine is impacted by faulty upstream turbine'.
2024-12-02 11:23:51 Faulty measurements for WTG 03 increased from 0.000 % to 0.000 %. Reason: 'Turbine is impacted by faulty upstream turbine'.
2024-12-02 11:23:51 Faulty measurements for WTG 04 increased from 0.000 % to 0.000 %. Reason: 'Turbine is impacted by faulty upstream turbine'.
2024-12-02 11:23:51 Faulty measurements for WTG 05 increased from 0.000 % to 0.000 %. Reason: 'Turbine is impacted by faulty upstream turbine'.
2024-12-02 11:23:51 Faulty measurements for WTG 06 increased from 0.000 % to 0.000 %. Reason: 'Turbine is impacted by faulty upstream turbine'.
0 1 2 3 4 5 6
wd
0.0 [6] [5] [3] [] [] [] []
3.0 [6] [5] [3] [] [] [] []
6.0 [6] [5] [3] [] [] [] []
9.0 [6] [5] [3] [] [] [] []
12.0 [] [5] [3] [] [] [] []
... ... ... ... .. .. .. ..
345.0 [6, 5] [5] [3] [] [] [] []
348.0 [6, 5] [5] [3, 5] [] [] [] []
351.0 [6] [5] [3] [] [] [] []
354.0 [6] [5] [3] [] [] [] []
357.0 [6] [5] [3, 5] [] [] [] []
[120 rows x 7 columns]
Show the final yaw angles#
# Show the effects of homogenization
# Show the wd channels for the turbines
fig, ax = plt.subplots(figsize=(12, 6))
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_001"],
label="wd_001 (Fixed Bias)",
color="blue",
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_002"],
label="wd_002 (Bias Changes)",
color="red",
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_000"],
label="wd_000",
color="k",
alpha=0.5,
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_003"],
label="wd_003",
color="k",
alpha=0.5,
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_004"],
label="wd_004",
color="k",
alpha=0.5,
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_005"],
label="wd_005",
color="k",
alpha=0.5,
)
ax.plot(
df_scada_homogenized["time"],
df_scada_homogenized["wd_006"],
label="wd_006",
color="k",
alpha=0.5,
)
ax.legend()
ax.set_xlabel("Time")
ax.set_ylabel("Wind direction (deg)")
Text(0, 0.5, 'Wind direction (deg)')
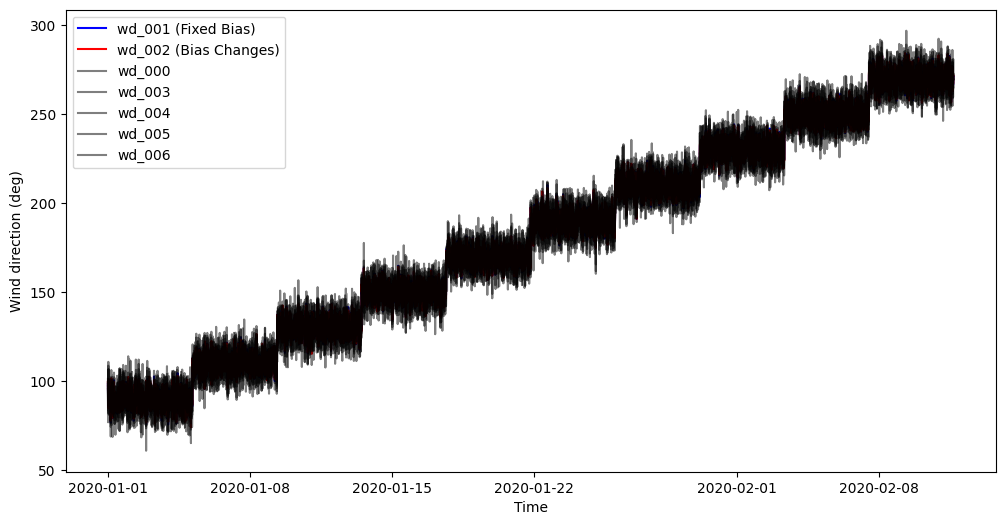